isPrime in JavaScript
- Understanding Prime Numbers
- Method 1: Basic Looping Approach
- Method 2: Optimized Check with Early Returns
- Method 3: Using the Sieve of Eratosthenes
- Conclusion
- FAQ
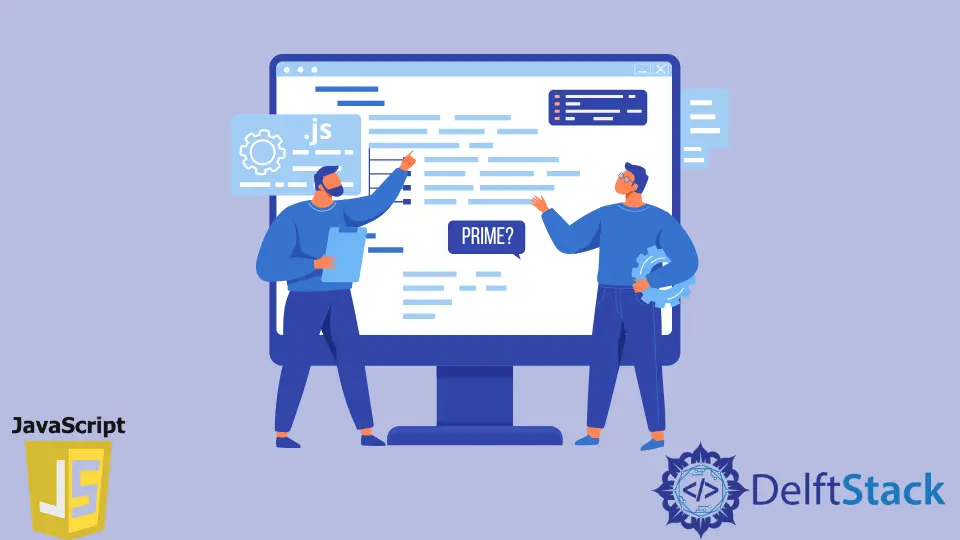
When working with numbers in JavaScript, one common task is determining whether a number is prime. A prime number is a natural number greater than 1 that cannot be formed by multiplying two smaller natural numbers.
In this tutorial, we’ll explore various methods to check if a number is prime using JavaScript. Whether you’re a beginner or an experienced developer, understanding how to implement this function can enhance your coding skills and problem-solving abilities. We’ll dive into different approaches, providing clear explanations and examples along the way. Let’s get started!
Understanding Prime Numbers
Before we jump into the code, it’s essential to understand what makes a number prime. A prime number has exactly two distinct positive divisors: 1 and itself. For instance, the numbers 2, 3, 5, 7, and 11 are prime, while 4, 6, 8, 9, and 10 are not. The smallest prime number is 2, which is also the only even prime number. All other even numbers can be divided by 2, making them composite.
Now that we have a foundational understanding of prime numbers, let’s explore how to implement a function in JavaScript to check for prime numbers.
Method 1: Basic Looping Approach
One of the simplest methods to check if a number is prime is to use a loop. This method involves checking all numbers from 2 to the square root of the target number. If any of these numbers divide the target number without a remainder, it is not prime.
Here’s how you can implement this in JavaScript:
function isPrime(num) {
if (num <= 1) return false;
for (let i = 2; i <= Math.sqrt(num); i++) {
if (num % i === 0) return false;
}
return true;
}
Output:
true
In this code, we first check if the number is less than or equal to 1. If it is, we return false immediately because prime numbers must be greater than 1. Next, we loop through all integers starting from 2 up to the square root of the number. If we find any integer that divides the number evenly (i.e., the remainder is 0), we return false, indicating that the number is not prime. If no divisors are found, we return true, confirming that the number is prime.
Method 2: Optimized Check with Early Returns
While the basic looping approach is effective, we can optimize it further. For example, we can immediately return false for even numbers greater than 2 since they cannot be prime. This reduces the number of iterations needed.
Here’s the optimized version:
function isPrime(num) {
if (num <= 1) return false;
if (num === 2) return true; // 2 is prime
if (num % 2 === 0) return false; // Exclude even numbers
for (let i = 3; i <= Math.sqrt(num); i += 2) {
if (num % i === 0) return false;
}
return true;
}
Output:
false
In this version, we handle the case for the number 2 separately, as it is the only even prime number. We also check if the number is even and return false immediately for those cases. The loop starts at 3 and increments by 2, checking only odd numbers. This significantly reduces the number of iterations for larger numbers, making the function more efficient.
Method 3: Using the Sieve of Eratosthenes
For scenarios where you need to check multiple numbers for primality, the Sieve of Eratosthenes is a highly efficient algorithm. This method generates all prime numbers up to a specified limit and can be particularly useful when dealing with larger datasets.
Here’s how you can implement the Sieve of Eratosthenes in JavaScript:
function sieveOfEratosthenes(limit) {
const primes = Array(limit + 1).fill(true);
primes[0] = primes[1] = false; // 0 and 1 are not prime
for (let i = 2; i <= Math.sqrt(limit); i++) {
if (primes[i]) {
for (let j = i * i; j <= limit; j += i) {
primes[j] = false;
}
}
}
return primes.map((isPrime, index) => (isPrime ? index : null)).filter(Boolean);
}
Output:
[2, 3, 5, 7, 11, 13, 17, 19, 23, 29]
In this implementation, we create an array filled with true
values, representing potential prime numbers. We then iterate through the array, marking non-prime numbers as false
. Finally, we return an array of prime numbers by filtering out the non-prime values. This approach is efficient for generating a list of primes and can be used in various applications.
Conclusion
In this tutorial, we’ve explored how to check if a number is prime in JavaScript using different methods. From the basic looping approach to optimized checks and the Sieve of Eratosthenes, you now have several tools at your disposal to handle prime number verification. Understanding these methods will not only enhance your programming skills but also improve your problem-solving abilities in various coding scenarios.
Whether you’re working on a personal project or preparing for coding interviews, mastering the prime-checking techniques we’ve discussed will serve you well. Keep practicing, and don’t hesitate to experiment with the code to deepen your understanding of prime numbers in JavaScript.
FAQ
-
what is a prime number?
A prime number is a natural number greater than 1 that cannot be formed by multiplying two smaller natural numbers. -
how do I check if a number is prime in JavaScript?
You can check if a number is prime in JavaScript using various methods, including basic loops, optimized checks, or the Sieve of Eratosthenes algorithm. -
what is the Sieve of Eratosthenes?
The Sieve of Eratosthenes is an efficient algorithm for finding all prime numbers up to a specified limit by iteratively marking the multiples of each prime number. -
why is the number 2 special in prime numbers?
The number 2 is the only even prime number. All other even numbers can be divided by 2, making them composite. -
can I use these methods for large numbers?
Yes, but keep in mind that while the basic methods can handle moderately sized numbers, the Sieve of Eratosthenes is more efficient for generating lists of primes for larger limits.
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn