How to Infinite Scroll in JavaScript
- What is Infinite Scrolling?
- Setting Up Your Project
- Fetching Data Dynamically
- Implementing Scroll Event
- Optimizing Performance
- Conclusion
- FAQ
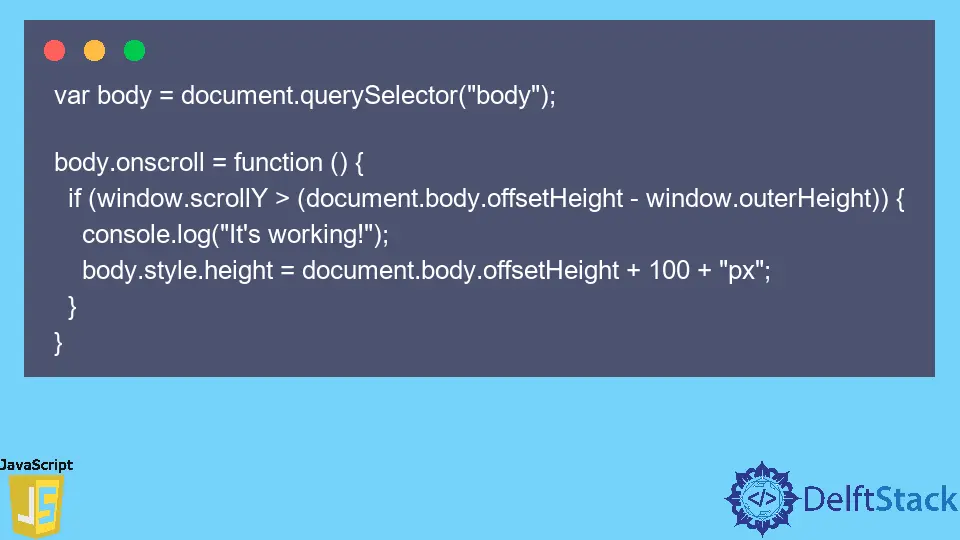
In today’s post, we’ll learn about infinite scrolling in JavaScript. Infinite scrolling is a web design technique that allows users to continuously load content as they scroll down a webpage, creating a seamless experience. This method is particularly popular in social media feeds, image galleries, and news sites, where users often want to explore more content without having to click through pages. By the end of this article, you’ll have a solid understanding of how to implement infinite scrolling in your JavaScript projects, enhancing user engagement and satisfaction. So, let’s dive in!
What is Infinite Scrolling?
Infinite scrolling is a web design pattern that loads content dynamically as users scroll down the page. Unlike traditional pagination, where users must click to navigate through pages, infinite scrolling automatically fetches new content, providing a smoother and more engaging user experience. This technique is especially useful for applications that display large sets of data, such as social media feeds or image galleries.
The primary goal of infinite scrolling is to keep users engaged by reducing the friction of clicking through multiple pages. However, it’s essential to implement it thoughtfully to ensure that users can easily navigate your site and find what they’re looking for.
Setting Up Your Project
To start implementing infinite scrolling in JavaScript, you first need to set up a simple HTML structure. Here’s a basic example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Infinite Scroll Example</title>
<style>
body { font-family: Arial, sans-serif; }
.content { margin: 20px; }
.item { border: 1px solid #ccc; padding: 10px; margin-bottom: 10px; }
</style>
</head>
<body>
<div class="content" id="content"></div>
<script src="script.js"></script>
</body>
</html>
This HTML file creates a simple layout with a content area where items will be displayed. The script.js
file will contain the JavaScript code for infinite scrolling.
Fetching Data Dynamically
The next step is to fetch data dynamically as the user scrolls down the page. For this example, we will simulate fetching data from an API. Here’s how you can do it:
let page = 1;
function fetchData() {
fetch(`https://jsonplaceholder.typicode.com/posts?_page=${page}&_limit=5`)
.then(response => response.json())
.then(data => {
const content = document.getElementById('content');
data.forEach(post => {
const div = document.createElement('div');
div.classList.add('item');
div.innerHTML = `<h2>${post.title}</h2><p>${post.body}</p>`;
content.appendChild(div);
});
page++;
})
.catch(error => console.error('Error fetching data:', error));
}
fetchData();
In this code, we define a fetchData
function that retrieves posts from a placeholder API. The page
variable keeps track of the current page to fetch. Each time the function is called, it appends new posts to the content area. The catch
block handles any errors that may occur during the fetch operation.
Implementing Scroll Event
Now that we have the data fetching part, the next step is to implement a scroll event listener that triggers the data fetching as the user scrolls down the page. Here’s how to do it:
window.addEventListener('scroll', () => {
if (window.innerHeight + window.scrollY >= document.body.offsetHeight) {
fetchData();
}
});
Output:
This event listener checks if the user has scrolled to the bottom of the page and calls the fetchData function to load more content.
This code listens for the scroll event on the window. When the user scrolls to the bottom of the page, it calls the fetchData
function to load more content. This creates a continuous loading experience, allowing users to explore more posts without interruption.
Optimizing Performance
While infinite scrolling can enhance user experience, it’s essential to optimize performance to prevent potential issues. Here are some tips:
- Limit Data Fetching: Avoid fetching too much data at once. Instead, load a manageable number of items with each scroll.
- Debounce Scroll Events: Use a debounce function to limit how often the scroll event triggers the fetch function. This helps reduce unnecessary API calls.
- Loading Indicators: Consider adding loading indicators to inform users that new content is being fetched, enhancing the user experience.
Implementing these optimizations can help ensure that your infinite scrolling feature runs smoothly and efficiently.
Conclusion
In this article, we explored how to implement infinite scrolling in JavaScript. We covered the basics of setting up your project, fetching data dynamically, and implementing a scroll event listener. With these techniques, you can create a seamless experience for users, allowing them to explore content without interruptions. Remember to optimize your implementation for performance to ensure a smooth experience. Happy coding!
FAQ
-
What is infinite scrolling?
Infinite scrolling is a web design technique that automatically loads more content as users scroll down a webpage, eliminating the need for pagination. -
How can I implement infinite scrolling in JavaScript?
You can implement infinite scrolling by fetching data dynamically using JavaScript and listening for scroll events to load more content automatically. -
What are some best practices for infinite scrolling?
Best practices include limiting data fetching, debouncing scroll events, and providing loading indicators to enhance user experience. -
Can I use infinite scrolling on mobile devices?
Yes, infinite scrolling can be effectively used on mobile devices, but be mindful of performance and usability. -
How do I handle errors when fetching data for infinite scrolling?
Implement error handling in your fetch function to manage any issues that may arise during data retrieval.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn