How to Get HTTP GET Request in JavaScript
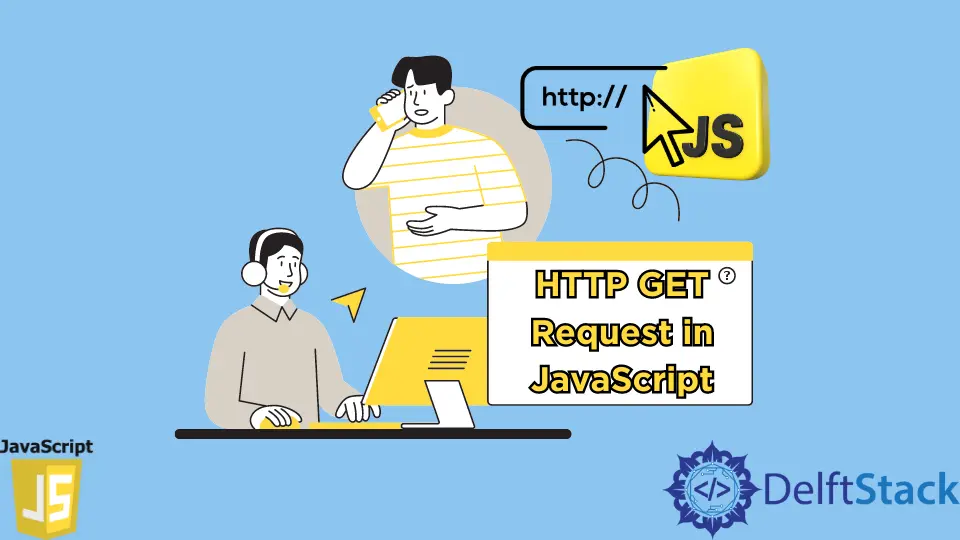
In JavaScript, we usually use the XMLHttpRequest
API to transfer data between web servers and browsers via its methods. Recently, the floor has been owned by the Fetch
API as it has easy implementation and promises enabled.
Additionally, the Fetch
convention supports the ES6 updates and modifications.
Here, we will demonstrate the instances that only use the XMLHttpRequest
API objects and the Fetch
API to grab the HTTP GET Request
from the server to the web browser.
Use the XMLHttpRequest
API to Retrieve GET
Request
Using the XMLHttpRequest
API, we will initialize an object named xmlhr
and launch the other methods available for this API.
Firstly, we will use the open
method to set the GET
from the server along with the URL
.
Also, we will see a false
parameter in the open
method, which is used in the case of synchronous requests true in the case of asynchronous requests
.
Code Snippet:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>Test</title>
</head>
<body>
<button onclick="httpGet('https://jsonplaceholder.typicode.com/todos/1')">Get</button>
<p id="get"></p>
</body>
</html>
function httpGet(theUrl) {
var xmlhr = new XMLHttpRequest();
xmlhr.open('GET', theUrl, false);
xmlhr.send(null);
document.getElementById('get').innerHTML = xmlhr.responseText;
return xmlhr.responseText;
}
Output:
Use the fetch
API to Retrieve GET
Request
If you are looking for an easy and better performance method to extract data from the server, the fetch
API makes the process pretty convenient.
As you will see in the example, the commands for this API are more predictable, and easy to trace the method of work. Initially, you will fetch the URL
then detect the data type.
Later we will extract the data and check if there is any error available. Finally, the output will preview in the console panel if error-free. Let’s check the code fence for proper understanding.
Code Snippet:
fetch('https://jsonplaceholder.typicode.com/todos/1')
.then((r) => r.json())
.then((data) => console.log(data))
.catch((e) => console.log('error'));
Output: