The goto Statement in JavaScript
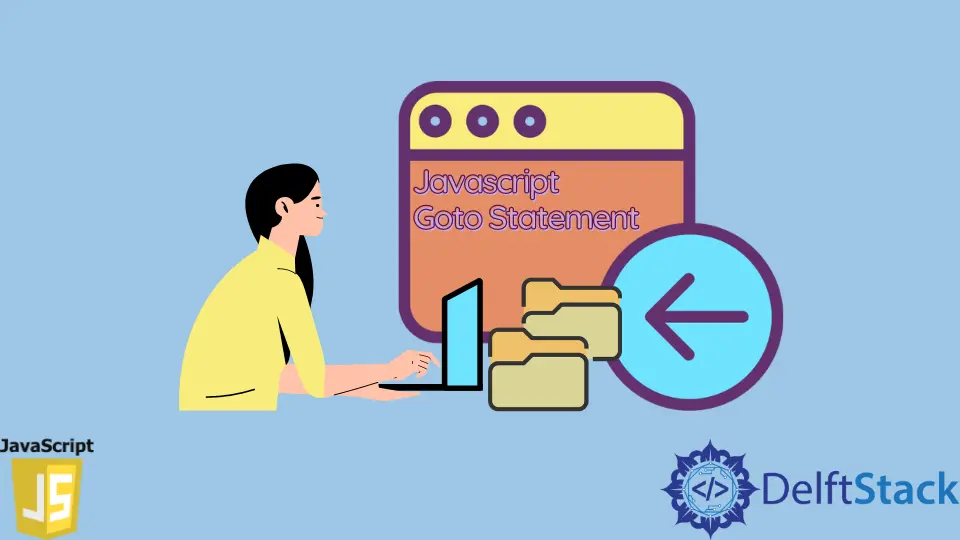
JavaScript doesn’t have any goto
keyword or method which can directly implement the goto
method. Reason being that it is in an unstructured manner, and goto
statement makes it hard to understand, and maintaining it is also tricky.
Still, we can use other ways to use the goto
statement indirectly in our code. We will discuss these methods in this tutorial.
The break
and continue
keywords can be utilized to imitate the goto
statement in JavaScript. The advantage of using these two methods is that it doesn’t have drawbacks like goto
.
The goto
keyword takes us to the user’s location if the conditions are True. The break
and continue
keywords together work as the goto
statement. The continue
statement will force the next iteration, and the break
statement will force the control out of the loop.
Let’s see an example of break
and continue
. Suppose we want to print some numbers that the user wants. We will be printing only the numbers that the user wants to display as the output here. Let’s say we take a variable x
and a loop for printing the required numbers.
See the code below.
javascriptCopyvar x;
for (x = 1; x <= 5; x++) {
if (x === 4 || x === 2) {
continue;
}
console.log(x);
if (x === 5) {
break;
}
}
Output:
textCopy1
3
5
In the above example, we shifted the control of the program using the break
and continue
statements. Whenever 2 and 4 are encountered, we force the next iteration using the continue
keyword. We break out the loop when it reaches a value of 5 using the break
keyword.
Similarly, we can use these keywords in other ways to emulate the goto
statement in JavaScript and shift the control of the program.