How to Get Value From JSON Object in JavaScript
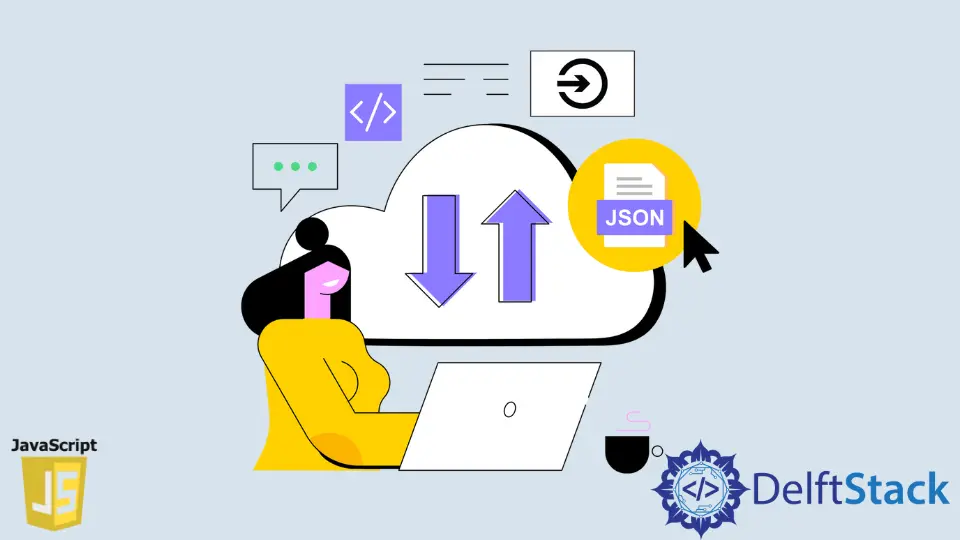
When data are received from the web-server, the format is in JSON (data-interchange format for web apps). The format previews the data in a key:value
pair and starts and ends with {}
(for object) or []
(for arrays). Keys are always tend to be string and values can be string and other data-types also.
This article will introduce how to get value from a JSON object in JavaScript.
Parse JSON Object in JavaScript With the JSON.parse()
Method
Let us consider the following JSON object as the received data.
{
"employee": {
"name": "Roy Mustang",
"age": 35,
"favoriteColor": ["Blue", "Black", "Purple"],
"siblings": {
"Liza": 20, "Emily": 12
}
}
}
The following code segment and image demonstrates the parsing method.
Code Snippet:
const json = `{
"employee": {
"name": "Roy Mustang",
"age": 35,
"favoriteColor": ["Blue", "Black", "Purple"],
"siblings": {
"Liza": 20, "Emily": 12
}
}
}`;
var data = JSON.parse(json);
var i;
for (i in data) {
if (data[i] instanceof Object) {
console.log(data[i]);
}
}
Output:
Access Individual JSON Data With Dot (.)
Operation in JavaScript
As we have already parsed the data, now let’s extract the individual values for key:value
pair with the dot (.
) operation.
Code Snippet:
const json = `{
"employee": {
"name": "Roy Mustang",
"age": 35,
"favoriteColor": ["Blue", "Black", "Purple"],
"siblings": {
"Liza": 20, "Emily": 12
}
}
}`;
var data = JSON.parse(json);
console.log(data.employee.name);
console.log(data.employee.favoriteColor[2]);
console.log(data.employee.siblings);
console.log(data.employee.siblings.Liza);
Output:
Access Individual JSON Data With Brackets []
in JavaScript
This convention allows you to retrieve data in a similar way like and array accessing its data.
Code Snippet:
const json = `{
"employee": {
"name": "Roy Mustang",
"age": 35,
"favoriteColor": ["Blue", "Black", "Purple"],
"siblings": {
"Liza": 20, "Emily": 12
}
}
}`;
var data = JSON.parse(json);
console.log(data['employee']['name']);
console.log(data['employee']['favoriteColor'][2]);
console.log(data['employee']['siblings']);
console.log(data['employee']['siblings']['Emily']);
Output:
Encode JSON Object in JavaScript
Supposedly, you are to modify a JSON object and resend back to server, or the task is to convert JavaScript to JSON format. This is often helpful as it is difficult to edit large sized JSON object. So, after parsing the object and redirecting it to the same format we use the JSON.stringify
method.
Code Snippet:
const json = `{
"employee": {
"name": "Roy Mustang",
"age": 35,
"favoriteColor": ["Blue", "Black", "Purple"],
"siblings": {
"Liza": 20, "Emily": 12
}
}
}`;
var data = JSON.parse(json);
data.employee.name = 'Riza Hawkeye';
const new_data = JSON.stringify(data);
console.log(new_data);
Output: