How to Get the Position of an Element Using JavaScript
-
Use the
Element.getBoundingClientRect()
Function to Get the Position of an Element in JavaScript -
Use the
offsetTop
Property to Get the Position of an Element in JavaScript
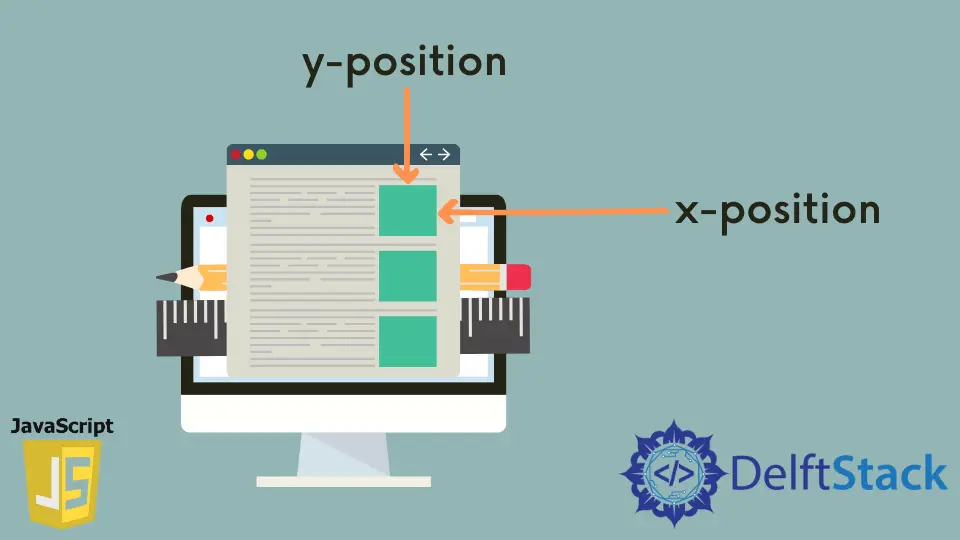
Every element in the HTML document is placed at a given position. The position here refers to the x and y coordinates of elements.
In this tutorial, we get the position of an HTML element in JavaScript.
Use the Element.getBoundingClientRect()
Function to Get the Position of an Element in JavaScript
The getBoundingClientRect()
function produces a DOMRect
object containing information about an element’s size and position in the viewport.
The DOMRect
object, when returned, contains a small rectangle that has the whole element, which includes the padding and the border-width.
The attributes such as the left
, top
, right
, bottom
, x
, y
, width
, and height
are used to define the total rectangle’s location, also size in pixels. The values obtained are relative to the viewports’ top-left corner, except for width and height.
For example,
let elem = document.querySelector('div');
let rect = elem.getBoundingClientRect();
console.log('x: ' + rect.x);
console.log('y: ' + rect.y);
Use the offsetTop
Property to Get the Position of an Element in JavaScript
It returns the top position relative to the top of the offsetParent
element. We can create a function that will return these coordinates.
For example,
function getOffset(el) {
var _x = 0;
var _y = 0;
while (el && !isNaN(el.offsetLeft) && !isNaN(el.offsetTop)) {
_x += el.offsetLeft - el.scrollLeft;
_y += el.offsetTop - el.scrollTop;
el = el.offsetParent;
}
return {top: _y, left: _x};
}
var x = getOffset(document.getElementById('div')).left;
var y = getOffset(document.getElementById('div')).top;
console.log('x: ' + x);
console.log('y: ' + y);