How to Get Element Value Using JavaScript
Kushank Singh
Feb 02, 2024
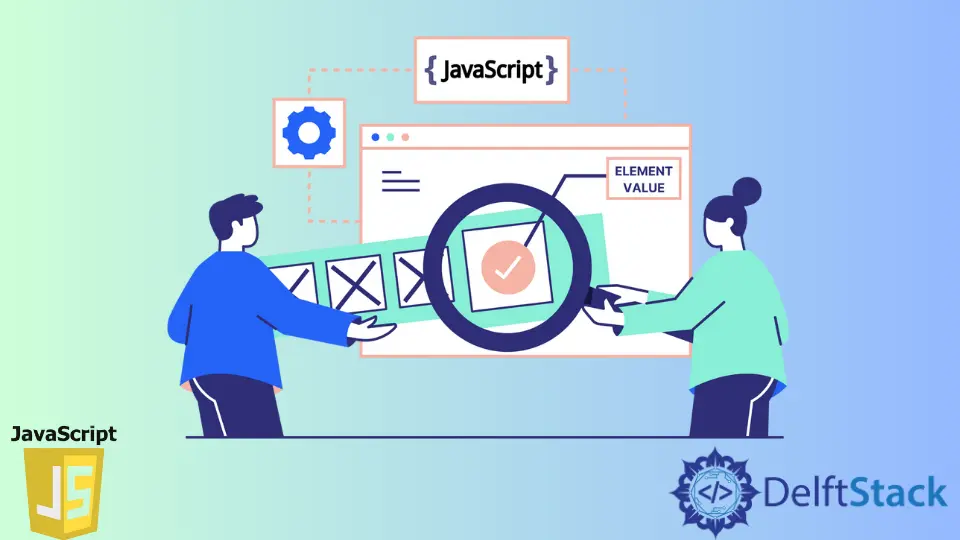
HTML webpages consist of different HTML elements, which have different attributes and values.
In this tutorial, we will get the value of an element using JavaScript.
To get the value of the element, we will use the value
property. It can be used to set or return the value of the value
attribute.
See the code below.
const variable = document.getElementsById(id).value;
In the above example, we first retrieve the required element using its ID with the getElementsById()
function. Then the value
property will return its value.
We can also select the element using the onclick
event handler in HTML. Then the element is passed onto the function, which retrieves its value using the required property.
For example,
// html
<input id = 'theId' value = 'test1' onclick = 'getValue(this)' />
// javascript
function getValue(e) {
const variable = e.value;
}