How to Get Element by Attribute in JavaScript
Kushank Singh
Feb 02, 2024
-
Use the
querySelectorAll()
Function to Get an Element by Its Attribute in JavaScript -
Use the
jQuery
Library to Get an Element by Its Attribute in JavaScript
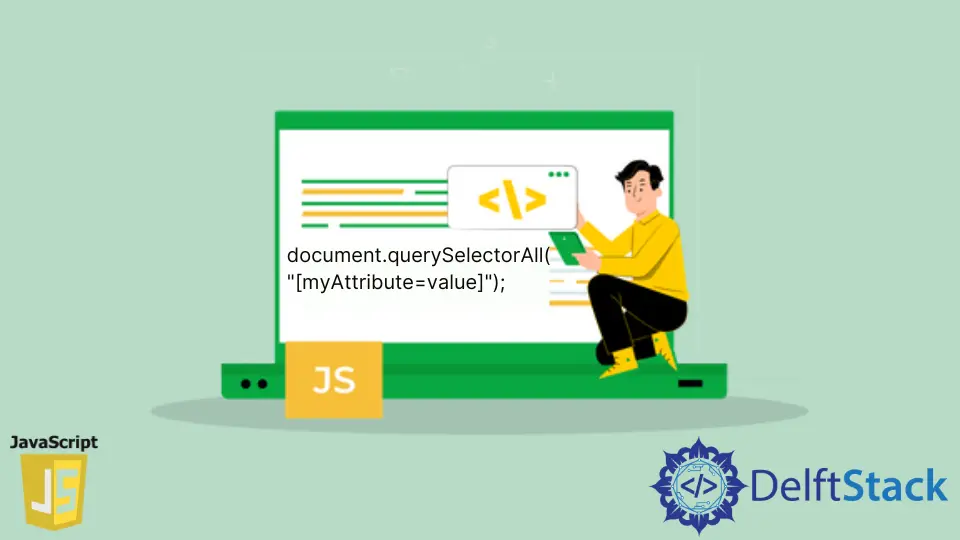
This tutorial will demonstrate how to get an element by its attribute in JavaScript.
Use the querySelectorAll()
Function to Get an Element by Its Attribute in JavaScript
The Document.querySelectorAll(selector)
method returns a list of the document elements that match the specified selectors. We can specify the attribute in the selectors.
Check the example below.
document.querySelectorAll('[myAttribute=value]');
The above example has an attribute selector, where the attribute value is defined, myAttribute=value
.
This function is supported with most modern browsers.
Use the jQuery
Library to Get an Element by Its Attribute in JavaScript
jQuery
is a fast, robust JavaScript library. We can select elements by their attribute using the jQuery
element selector $
.
For example,
$('[myAttribute=value]');
This method is valid with obsolete browsers also that support the jQuery
library.