How to Get Class Name in JavaScript
-
Use the
instanceof
Operator to Get the Class Name in JavaScript -
Use the
name
Property to Get the Class Name in JavaScript -
Use the
typeof
Operator to Get the Class Name in JavaScript -
Use the
isPrototypeOf()
Function to Get the Class Name in JavaScript
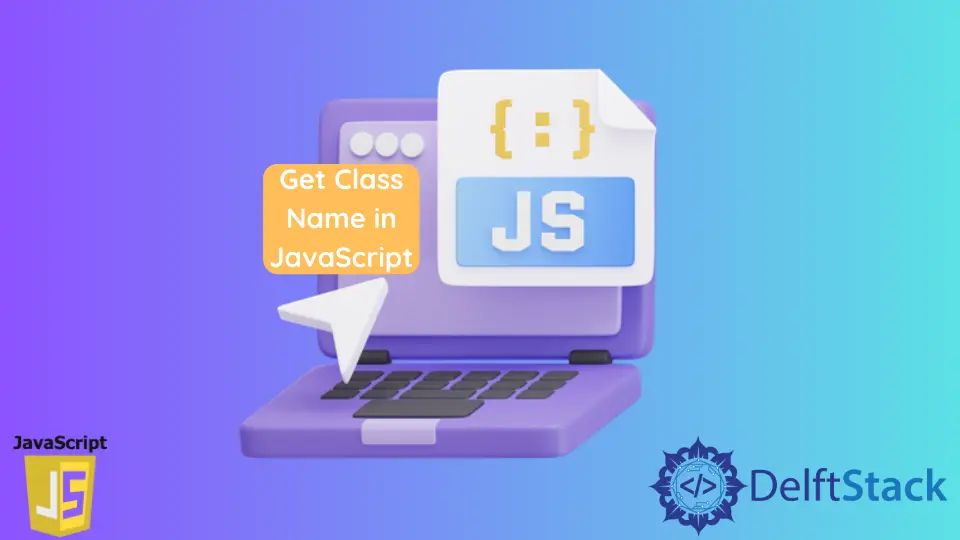
In JavaScript, you may need to get the name of a class from time to time. This is useful when utilizing the class name as an identifier or for debug messages.
In JavaScript, there is no equivalent to Java’s getClass()
function because Java is a class-based language, whereas JavaScript is a prototype-based language.
In this tutorial, we will get the class name in JavaScript.
Use the instanceof
Operator to Get the Class Name in JavaScript
The instanceof
operator does not directly give the class name but can check if the constructor’s prototype property occurs anywhere in the object’s prototype chain.
For example,
function Test() {}
let test = new Test();
console.log(test instanceof Test);
Output:
true
In the above example, test
belongs to Test
, and that is why it returns true
.
Use the name
Property to Get the Class Name in JavaScript
We can use the name
property of the object’s constructor to know the class name in JavaScript. This way, we get the name of that class through which we have instantiated the object.
For Example,
function Test() {}
let test = new Test();
console.log(test.constructor.name);
console.log(Test.name);
Output:
Test
Test
Use the typeof
Operator to Get the Class Name in JavaScript
The typeof
operator returns a string that indicates the type of the operand.
For Example,
function Test() {}
let test = new Test();
console.log(typeof Test);
console.log(typeof test);
Output:
function
object
Use the isPrototypeOf()
Function to Get the Class Name in JavaScript
The function isPrototypeOf()
function determines whether an object is another object’s prototype. First, we need to use the prototype
property of the object.
See the following example,
function Test() {}
let test = new Test();
console.log(Test.prototype.isPrototypeOf(test));
Output:
true