How to Get Child Element in JavaScript
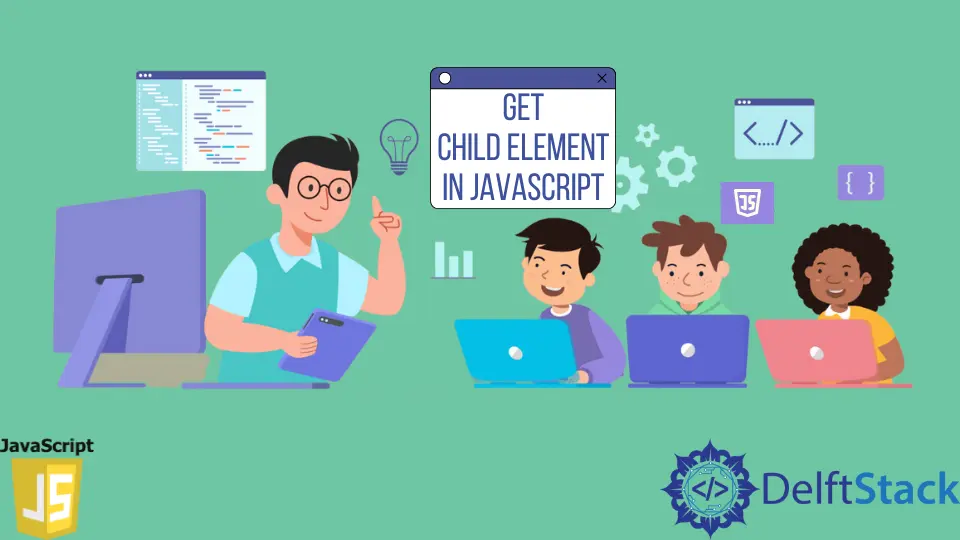
This article explains how to get the child nodes from the body of a web page in HTML using JavaScript.
Get the Child Nodes From the Body of a Web Page in HTML Using JavaScript
Getting a child of a node using JavaScript means accessing the whole page and getting it in an array. It’s important to remember that whitespaces are considered text nodes in the web page.
In the case of white spaces, the outdated browser treats it differently. Some of them are considered as childNodes
and others as children like Internet Explorer lesser than version 8.
<!DOCTYPE html>
<html>
<body>
<!-- we are writing a comment node! -->
<p>Click GET NODES button to get child nodes.</p>
<button onclick="getMyWebPageChildNodes()">GET NODES</button>
<p id="results"></p>
<script>
function getMyWebPageChildNodes() {
var myChildsNode = document.body.childNodes;
var textMessages = "";
var i;
for (i = 0; i < myChildsNode.length; i++) {
textMessages = textMessages + myChildsNode[i].nodeName + "<br>";
}
document.getElementById("results").innerHTML = textMessages;
}
</script>
</body>
</html>
In the above code, we create a button and set a listener on that button named onClick
.
We put an action on the onclick
named getMyWebPageChildNodes
. We created an array named myChildsNode
and stored all the child nodes in this function.
After that, we create a variable textMessages
in which we store the nodes purely without comments. A counter
variable is created to iterate the myChildsNode
array.
We made a for
loop in which we fetched the name of all nodes present on our web page and then stored it in the textMessages
variable. Once it’s done, you’ll get all the node names, and the for
loop will terminate.
Here we assign these nodes to our paragraph tag id=results
. Finally, we get all the children nodes in a paragraph tag.