How to Get Values of Data Attributes in JavaScript
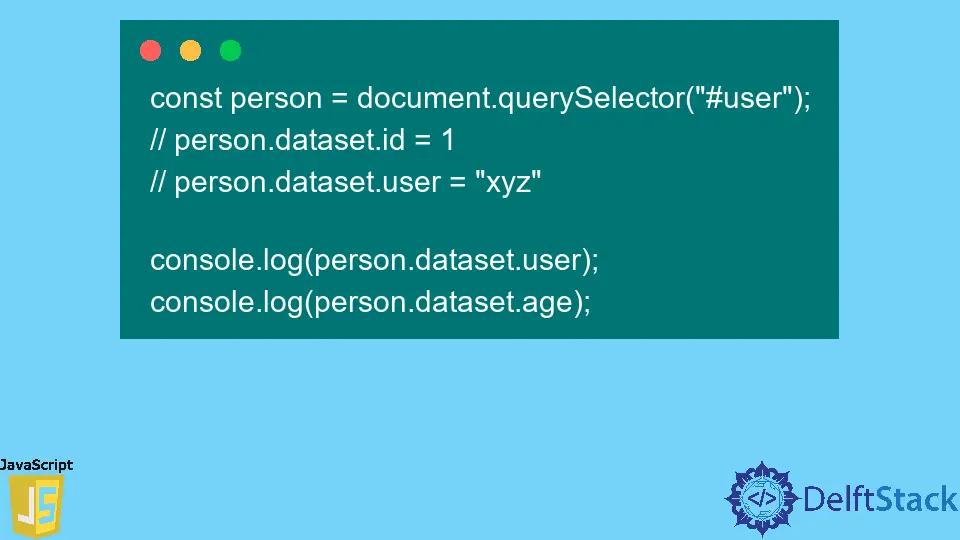
Attributes can be thought of as properties for HTML tags. Different HTML elements have different attributes, which are specified within the HTML element tag.
We will learn how to get values of data attributes in JavaScript in this article.
The dataset
property is a read-only property of the HTML element that helps us read/write data attributes. It gives a string map (DOMStringMap
) with the entry for every data-*
attribute. The name of the attribute should begin with data-
.
See the example below.
- HTML
<div id="user" data-id="1" data-user="xyz" data-age="18">User 1</div>
- JavaScript
To access the attribute name, remove the prefix data-
and convert dash-style
to camelCase
.
const person = document.querySelector('#user');
// person.dataset.id = 1
// person.dataset.user = "xyz"
console.log(person.dataset.user);
console.log(person.dataset.age);
Output:
xyz
18
In the above example, we select the required HTML element using the querySelector()
function. We access the data of the attributes using the dataset
property and print these accessed values. We display the data-user
and data-age
attributes in the above example.