How to Get an Apostrophe in a String Using JavaScript
Mehvish Ashiq
Feb 02, 2024
-
Use the Double Quotes (
""
) to Get an Apostrophe in a String in JavaScript -
Use the Escape Character (
\
) to Get an Apostrophe in a String in JavaScript
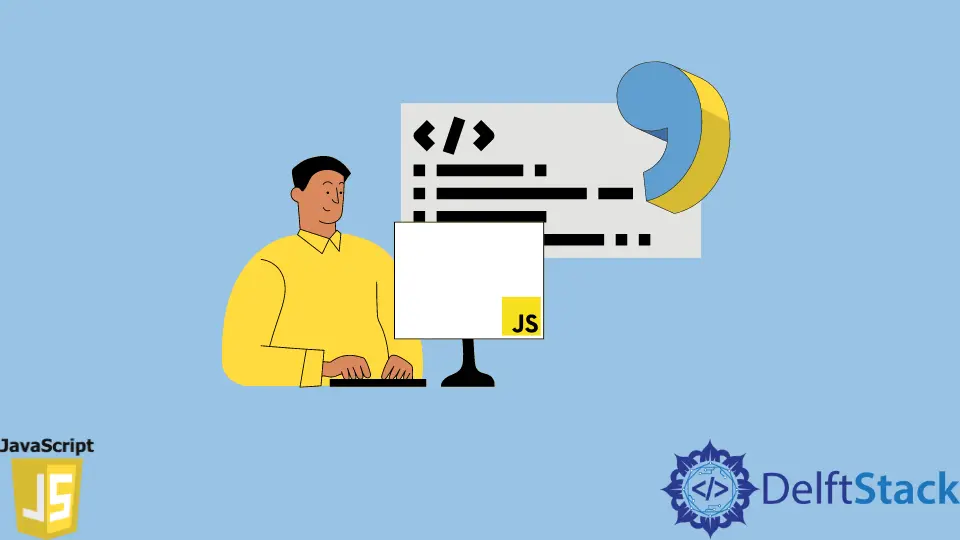
This tutorial explains how to get an apostrophe in a string using JavaScript by enclosing the string with single and double quotes.
Use the Double Quotes (""
) to Get an Apostrophe in a String in JavaScript
var message = 'Hi, Let\'s start reading if you are free!';
console.log(message);
Output:
"Hi, Let's start reading if you are free!"
Suppose the string has apostrophes on multiple positions in a string; can we still use double quotes? Yes, see the following example.
var message =
'I can start reading soon when I\'ll be free from this project, but it doesn\'t seem to happen.';
console.log(message);
Output:
"I can start reading soon when I'll be free from this project, but it doesn't seem to happen."
Use the Escape Character (\
) to Get an Apostrophe in a String in JavaScript
var message = 'Hi, Let\'s start reading if you are free!';
console.log(message);
Output:
"Hi, Let's start reading if you are free!"
You might think that if we are using an escape character, can we enclose the string in single quotes without affecting the results? Yes, of course.
var message = 'Hi, Let\'s start reading if you are free!';
console.log(message);
Output:
"Hi, Let's start reading if you are free!"
We can escape the apostrophe where ever it is needed in a string.
var message =
'I\'ll start reading soon when I\'ll be free from this project, but it doesn\'t seems to happen.';
console.log(message);
Output:
"I'll start reading soon when I'll be free from this project, but it doesn't seem to happen."
Author: Mehvish Ashiq