How to Generate Random Boolean in JavaScript
-
Use
Math.random()
to Generate Random Boolean in JavaScript - Use Array to Generate Random Boolean in JavaScript
-
Use the
_.sample(collection)
Method to Generate Random Boolean in JavaScript
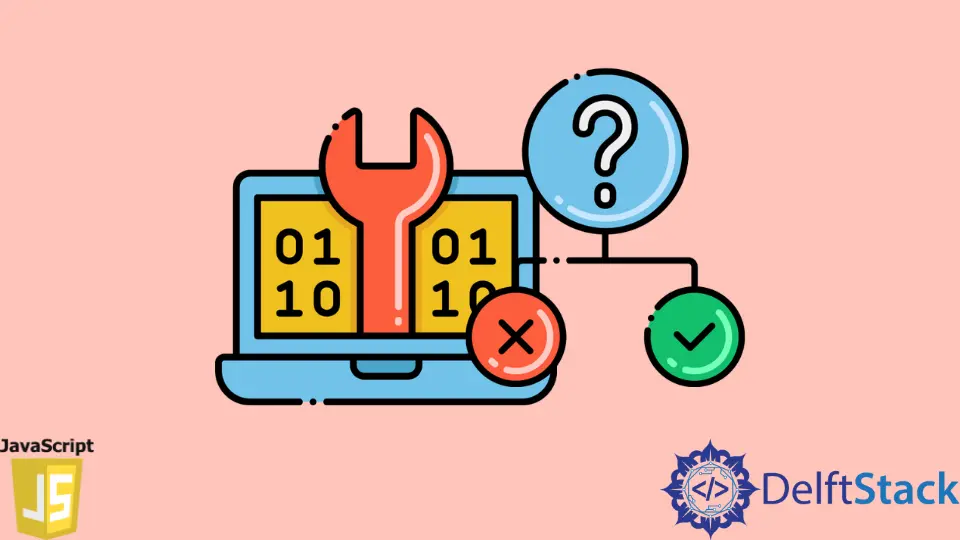
This article introduces how to generate random Boolean in JavaScript using Math.random()
, Arrays, and _.sample(collection)
function of lodash. The Math.random()
function returns the numbers between 0 and 1 where 0 is inclusive while 1 is exclusive.
The _.sample(collection)
method accepts a collection and returns one value randomly from the provided collection.
To use the _.sample(collection)
method of lodash, add the following line of code in the <head>
element.
<script src="https://cdn.jsdelivr.net/npm/lodash@4.17.10/lodash.min.js"></script>
Use Math.random()
to Generate Random Boolean in JavaScript
The Math.random()
returns any number in the range of [0,1)
where 0 is inclusive, but 1 is exclusive.
We can split this range into two partitions. First partition ranges from [0,0.5)
and second from [0.5,1)
.
Why are we doing this? Because we are giving an equal chance of occurrence to true
and false
. If it is less than 0.5, then true
; otherwise, the Boolean value would be false
.
Example:
var random_boolean_value = Math.random() < 0.5;
console.log(random_boolean_value)
Output:
false
Let’s write a more detailed code to see that it returns true
if less than 0.5.
See the following snippet.
for (var i = 0; i < 10; i++) {
var rand_number = Math.random();
console.log(rand_number);
var random_boolean_value = rand_number < 0.5;
console.log(random_boolean_value);
}
Output:
0.06464303463834886
true
0.27911502950509837
true
0.9811371177556913
false
0.539086724802587
false
0.3440647317306955
true
0.8779878853066467
false
0.2889025142115962
true
0.6346333079296975
false
0.28944321051370525
true
0.35970422088985354
true
Use Array to Generate Random Boolean in JavaScript
Let’s learn a more optimized version to generate random Boolean in JavaScript.
Check the following instance where we use Math.random()
to produce a random value, multiplied by Array.prototype.length
. Then, use the Math.floor()
method to round off the nearest whole number.
Example:
const sample = arr => arr[Math.floor(Math.random() * arr.length)];
console.log(sample([true, false]));
Output:
true
Use the _.sample(collection)
Method to Generate Random Boolean in JavaScript
Example:
<html>
<head>
<script src="https://cdn.jsdelivr.net/npm/lodash@4.17.10/lodash.min.js"></script>
</head>
<body>
<script>
console.log(_.sample([true, false]));
</script>
</body>
</html>
Output:
false