Nested Functions in JavaScript
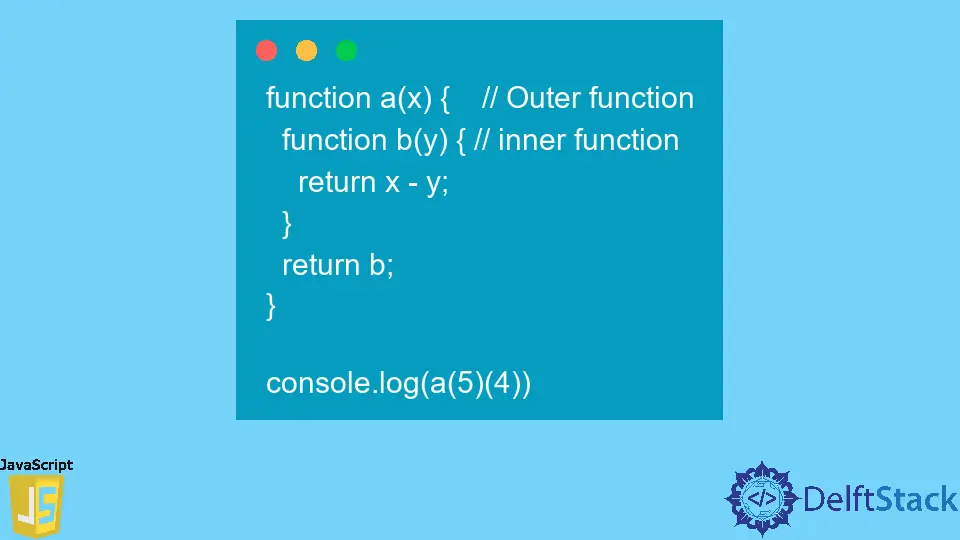
Functions are a useful block of code that can be called anywhere required in a program. A nested function is a function within a function. Such a feature is supported in many programming languages, JavaScript included.
We will introduce nested functions in JavaScript in this tutorial.
The function on the outside is termed the outer function. The function nested inside is called the inner function. Each function can accept different arguments.
They can be implemented in the following way.
function a(x) { // Outer function
function b(y) { // inner function
return x - y;
}
return b;
}
console.log(a(5)(4))
Output:
1
In the above example, a()
is the outer function, and b()
is the inner function. The final result returned uses the arguments from both the functions.
Functions are class objects that can be defined in the outer function and created like a variable at any part of the function. This method is called currying.
See the code below.
function outer(x) {
var w = function inner(y) {
return x * y;
} return w;
};
var outvar = outer(2);
console.log(outvar(4));
Output:
8
The nested functions have another benefit to it. They can be used to perform calculations inside another function, even if they are defined outside.
For Example,
function calculate(a, b, fn) {
var c = a + b + fn(a, b);
return c;
}
function sum(a, b) {
return a + b;
}
function product(a, b) {
return a * b;
}
console.log(calculate(10, 20, sum));
console.log(calculate(10, 20, product));
Output:
60
230