How to Get Full-Screen Window in JavaScript
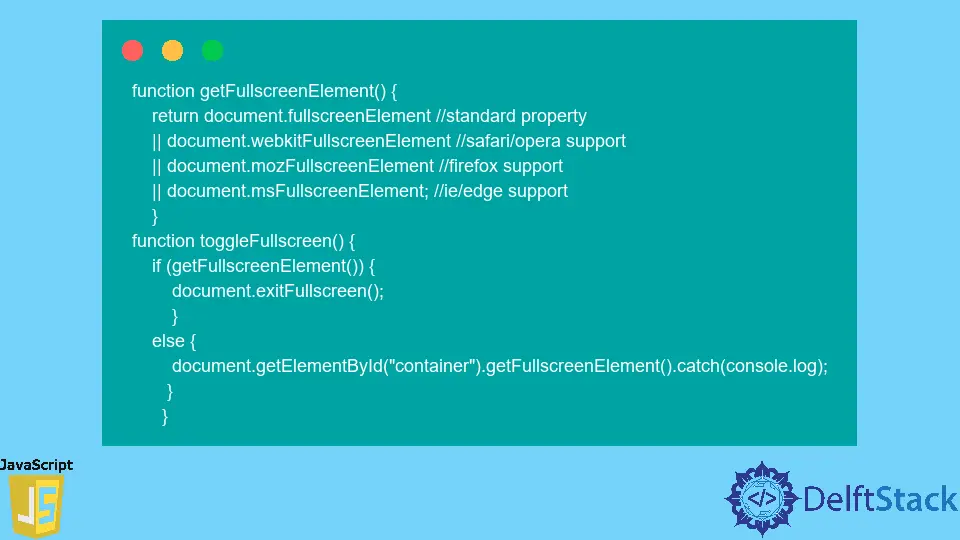
In this tutorial, we will learn how to get a full-screen window using JavaScript.
The full-screen API helps us use JavaScript code to convert a web page to full-screen mode. We can apply it to either the whole document or an individual element.
Check the example below.
function getFullscreenElement() {
return document.fullscreenElement // standard property
|| document.webkitFullscreenElement // safari/opera support
|| document.mozFullscreenElement // firefox support
|| document.msFullscreenElement; // ie/edge support
}
function toggleFullscreen() {
if (getFullscreenElement()) {
document.exitFullscreen();
} else {
document.getElementById('container')
.getFullscreenElement()
.catch(console.log);
}
}
In the code above, There are four different options in the getFullscreenElement()
function such as document.fullscreenElement
, document.webkitFullscreenElement
, document.mozFullscreenElement
, and document.msFullscreenElement
, which makes full-screen command compatible with almost every browser.
The toggleFullscreen()
makes the window enter into a full-screen mode which is not in full-screen mode, and vice-versa by clicking on the Enter Full-Screen button. We can call this function on given elements in the following way.
document.getElementById('button').addEventListener('click', () => {
toggleFullscreen();