How to Calculate the Median in JavaScript
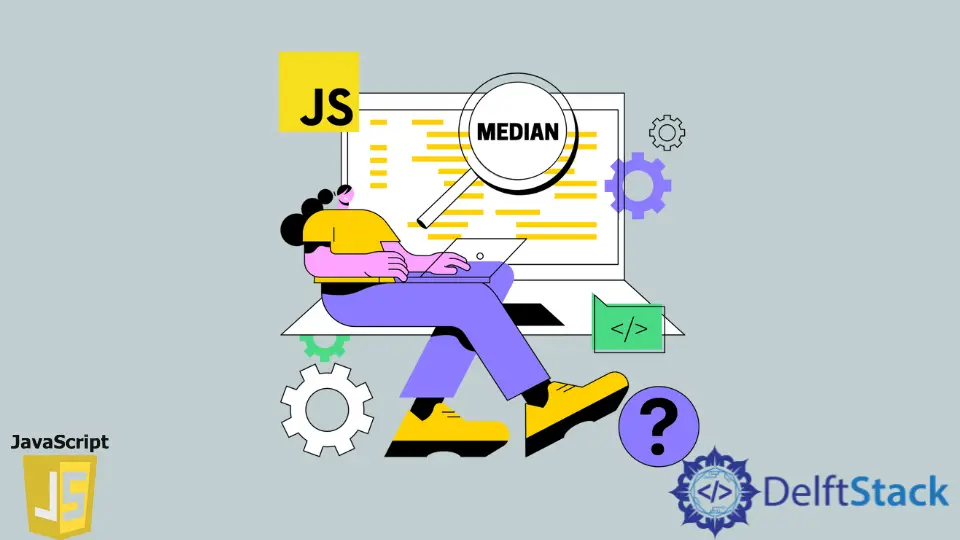
To get the median value in JavaScript, sort an array of integers using the sort()
method. Then return the number at the midpoint if the length is odd, or the average of the two middle values in the array if the length is even.
Get the Median Value in JavaScript
The middle number in the sorted array of numbers is called median. The median will be selected based on the number of elements in the array.
If the array’s length is odd
, the middle element is selected as the median.
list = [1, 2, 3]
len = 3
middleIndex = floor(len / 2); // 1
median = list[middleIndex]; // 2
If the length of the list is an even value, then the middle element is selected by adding the middle element with the previous element and dividing the result by 2.
list = [1, 2, 3, 4]
len = 4
middleIndex = floor(len / 2); // 2
median = (list[middleIndex] + list[middleIndex - 1]) / 2; // (3+2)/2 -> 2.5
median; // 2.5
Below the sample is a snippet of getting the median of an array of numbers.
First, we create the getSortedArray()
function to create a new sorted array from the input array and return it. Then, create a function findMedian()
that will return the median of an array.
Example:
function getSortedArray(arr) {
return arr.slice().sort((a, b) => a - b);
}
function findMedian(inputArray) {
let sortedArr = getSortedArray(inputArray);
let inputLength = inputArray.length;
let middleIndex = Math.floor(inputLength / 2);
let oddLength = inputLength % 2 != 0;
let median;
if (oddLength) { // if array length is odd -> return element at middleIndex
median = sortedArr[middleIndex];
} else {
median = (sortedArr[middleIndex] + sortedArr[middleIndex - 1]) / 2;
}
return median;
}
Output:
"findMedian([1,2,3]) => " 2
"findMedian([1,2,3,4,5,6]) => " 3.5