How to Escape Quotes in JavaScript
- Understanding the Basics of Escaping Quotes
- Using Entity Characters to Escape Quotes
- Combining Different Escape Methods
- Best Practices for Escaping Quotes in JavaScript
- Conclusion
- FAQ
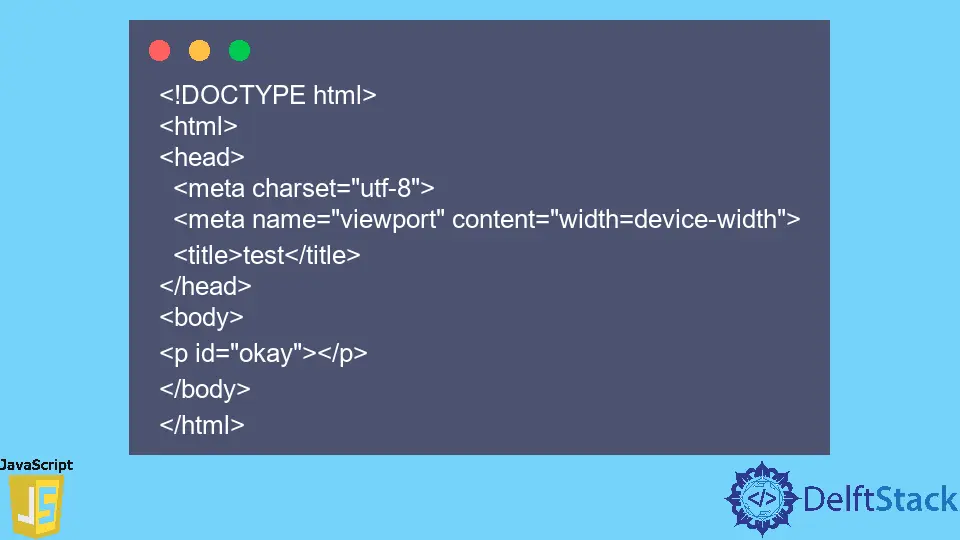
JavaScript is a powerful language that allows developers to manipulate text in various ways. One common challenge developers face is the need to escape quotes within strings. Whether you’re using single quotes, double quotes, or backslashes, understanding how to escape quotes is essential for writing clean and functional code.
In this article, we will explore the different methods for escaping quotes in JavaScript, including the use of entity characters. By the end, you’ll have a solid grasp of how to handle quotes effectively, ensuring your strings are always formatted correctly. Let’s dive in!
Understanding the Basics of Escaping Quotes
In JavaScript, quotes can be tricky, especially when you want to include them within a string. You can use both single quotes ('
) and double quotes ("
) to define strings. However, if you want to include a quote of the same type inside the string, you need to escape it. This is where the backslash (\
) comes into play.
For example, if you’re using single quotes to define a string and you want to include a single quote in that string, you would escape it like this:
let example = 'It\'s a sunny day';
Output:
It's a sunny day
In this case, the backslash tells JavaScript to treat the following single quote as a literal character rather than the end of the string. This method applies similarly to double quotes. If you wanted to include double quotes within a double-quoted string, you would do this:
let example = "He said, \"Hello!\"";
Output:
He said, "Hello!"
Using the backslash for escaping quotes is an essential skill for any JavaScript developer. It allows you to create strings that can contain quotes without breaking the syntax.
Using Entity Characters to Escape Quotes
Another effective way to escape quotes in JavaScript is by using HTML entity characters. This method is particularly useful when working with strings that will be displayed in a web browser. Entity characters are special codes that represent characters that might otherwise be interpreted as HTML code.
For example, instead of using a backslash to escape a double quote, you can use the entity character "
. Here’s how you can do that:
let example = "He said, "Hello!"";
Output:
He said, "Hello!"
This method can be beneficial when you’re dealing with HTML content and want to ensure that your quotes are displayed correctly. It helps prevent issues with rendering and ensures that your strings remain intact.
Using entity characters is a good practice when working with web applications, as it enhances the robustness of your code. It also makes your code cleaner and easier to read, especially for those who may not be familiar with escaping characters.
Combining Different Escape Methods
You may find situations where you need to combine different escape methods to achieve your desired outcome. JavaScript allows you to mix and match single quotes, double quotes, and backslashes, giving you flexibility in how you construct your strings.
For instance, consider the following example where you want to include both single and double quotes in a string:
let example = 'She said, "It\'s a beautiful day!"';
Output:
She said, "It's a beautiful day!"
In this case, you can see that the single quote is escaped with a backslash, while the double quotes are used without any escaping. This flexibility allows you to create complex strings that include various types of quotes.
Another example could be using backslashes to escape backslashes themselves. This can be particularly useful when working with file paths or regular expressions:
let example = "This is a backslash: \\";
Output:
This is a backslash: \
By mastering the combination of different escape methods, you can craft strings that meet all your needs without running into syntax errors.
Best Practices for Escaping Quotes in JavaScript
While escaping quotes is a straightforward task, adhering to best practices can make your code more maintainable and less error-prone. Here are a few tips to keep in mind:
-
Consistency: Choose either single or double quotes for your strings and stick to it throughout your code. This consistency helps avoid confusion and makes your code easier to read.
-
Use Template Literals: ES6 introduced template literals, which allow you to use backticks (
`
) to create strings. This method supports multi-line strings and eliminates the need for escaping quotes altogether. For example:let example = `He said, "It's a beautiful day!"`;
Output:
He said, "It's a beautiful day!"
-
Minimize Escaping: Whenever possible, structure your strings to minimize the need for escaping. For example, if you need to include a lot of quotes, consider using template literals or alternating between single and double quotes.
By following these best practices, you’ll not only make your code cleaner but also reduce the likelihood of bugs related to improperly escaped quotes.
Conclusion
Escaping quotes in JavaScript is an essential skill for any developer. Whether you’re using backslashes or entity characters, understanding how to handle quotes effectively can save you from syntax errors and make your code more robust. Remember to practice consistency and consider using template literals to simplify your string handling. With these techniques in your toolkit, you’ll be well-equipped to manage quotes in any JavaScript project.
FAQ
-
What is the purpose of escaping quotes in JavaScript?
Escaping quotes allows you to include quote characters within strings without breaking the syntax. -
Can I use both single and double quotes in JavaScript strings?
Yes, you can use both single and double quotes. Just ensure you escape the type of quote you are using within the string. -
What are template literals in JavaScript?
Template literals are string literals enclosed by backticks that allow for multi-line strings and easier variable interpolation. -
Are there any performance differences between using escaped quotes and entity characters?
Generally, there are no significant performance differences, but using escaped quotes is often simpler and more straightforward.
- What should I do if I have too many quotes in a string?
Consider using template literals or restructuring your string to minimize the need for escaping.