How to Embed HTML in JavaScript
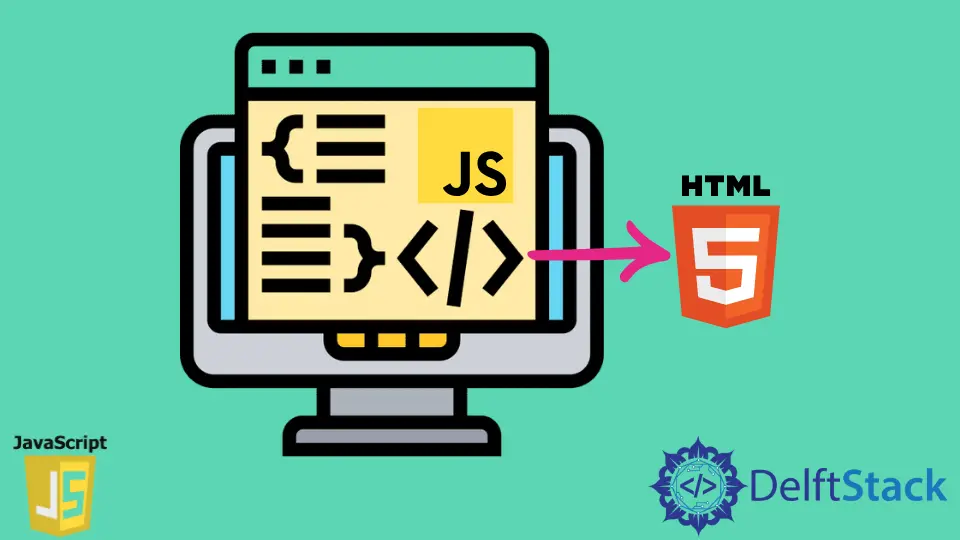
Hypertext Markup Language is at the root of all web pages displayed on the internet. The HTML DOM is updated automatically by changing the nodes of the DOM.
This can be done by updating the existing DOM structure or adding new nodes. In today’s post, we’ll learn about embedding HTML in JavaScript.
Embed HTML in JavaScript
The element’s innerHTML
property gets or sets the HTML or XML markup contained in the element.
To insert the HTML into the document, instead of replacing the content of an element, use the insertAdjacentHTML()
method.
Once the innerHTML
is set, all of the element’s descendants are removed. innerHTML
replaces them with nodes created by parsing the provided HTML into the htmlString
string.
It returns the HTML serialization of the element’s descendants as a string.
The following steps will be executed after setting innerHTML
in the background.
- The given value is parsed as either HTML or XML (depending on the document type), resulting in a
DocumentFragment
object representing the new set of DOM nodes for the new elements. - If the element whose content is being replaced is a template element, the content attribute of the template element is replaced with the new
DocumentFragment
created in step 1. - For all other elements, the element’s content is replaced with the nodes in the new
DocumentFragment
.
A SyntaxError
is thrown if an attempt was made to set the value of innerHTML
using a not properly-formed HTML string. You can find more information about the innerHTML
documentation for innerHTML
.
Let’s understand that with an example.
<div id="list-id"></div>
document.getElementById('list-id').innerHTML =
'<ol><li>html data</li><li>JS data</li></ol>';
In the above code, we have defined one div
tag. You can access the div
tag using getElementById
and append the ordered list by accessing the innerHTML
property of the div
tag.
Output:
1. html data
2. JS data
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn