How to Display Image With JavaScript
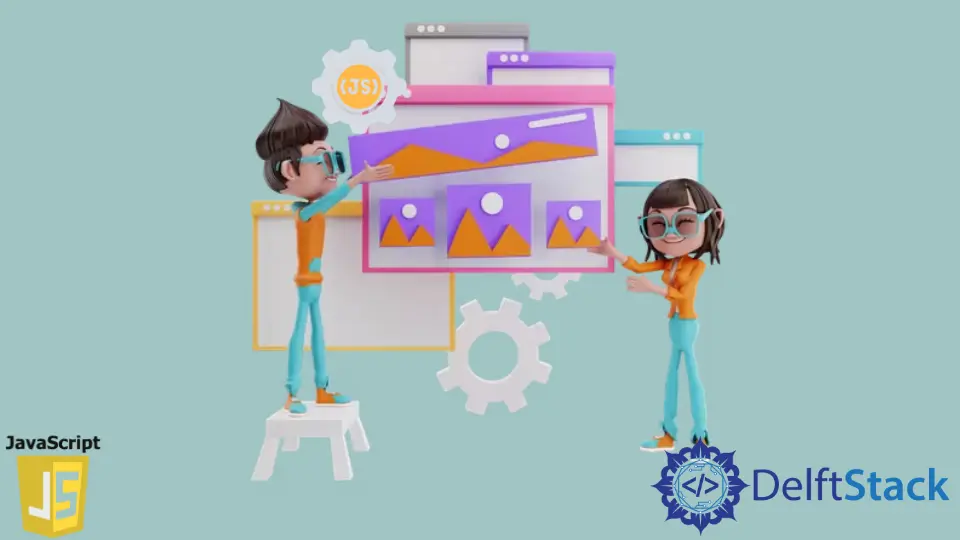
JavaScript is known as the web development language. By using JavaScript, we can make our web page attractive by inserting images into it.
By default, we use the <img>
tag in HTML to display images. In the <img>
tag, we have a method known as src
, which helps get the source of the image that we gave to display. In the src
, we have to give the complete path of the image; otherwise, it will raise an error. The width, height of the image also needs to be specified.
JavaScript has its own img
tag, which helps us insert an image in our webpage. We can create a reusable function. Here we will be using the img
element again but differently.
We will be creating a function called display_image()
, which will consist of the parameters like source
, width
, height
, and alt
of an image in it. We will be using a createElement()
for creating an element in the function. This way, we can dynamically display images wherever we call this function.
For example,
<!DOCTYPE html>
<html>
<body>
<p id="demo"></p>
<script>
function display_image(src, width, height, alt) {
var a = document.createElement("img");
a.src = src;
a.width = width;
a.height = height;
a.alt = alt;
document.body.appendChild(a);
}
display_image('JavaScript.jpg',
276,
110,
'JavaScriptImage');
</script>
</body>
</html>
Output:
The above example displays an image on the webpage based on the provided source. The document.body.appendChild()
function adds the given element to the body of the webpage.