How to Detect Mobile Browser in JavaScript
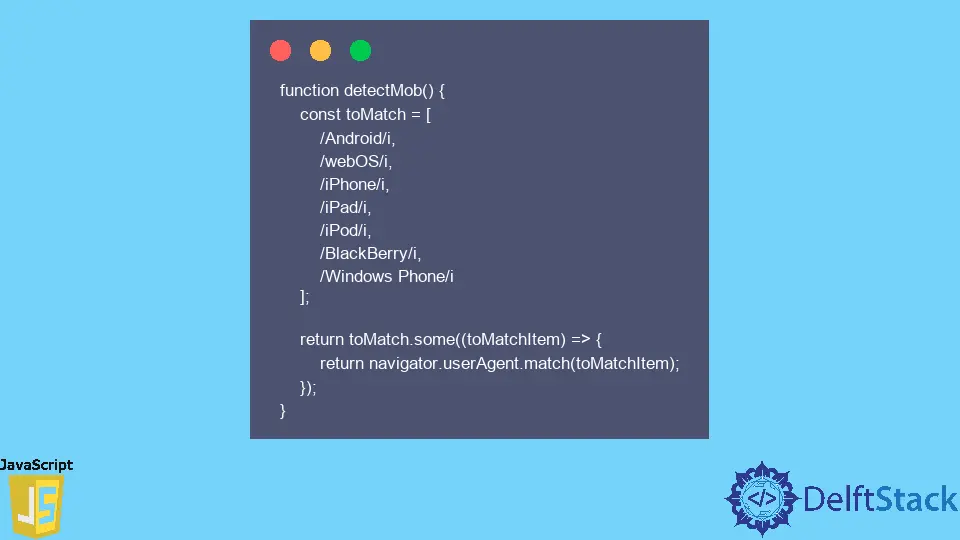
JavaScript is widely used for developing web-based applications. Most of these applications are also compatible with mobile browsers.
This tutorial will demonstrate the various methods available to detect a mobile browser using JavaScript.
Detect Mobile Browser by Detecting Device Used
The most simple and straightforward approach to identify a mobile browser using JavaScript is to run through a list of various devices available in the market today and check if the useragent
complements either one of the devices in the list available to us.
The following is the code snippet of the function which we’ll be working with -
function detectMob() {
const toMatch = [
/Android/i, /webOS/i, /iPhone/i, /iPad/i, /iPod/i, /BlackBerry/i,
/Windows Phone/i
];
return toMatch.some((toMatchItem) => {
return navigator.userAgent.match(toMatchItem);
});
}
We can further narrow down our search for mobile devices by assuming that the target device is mobile if the resolution is less than or equal to 800x600, even though most mobiles available today offer a much greater resolution.
Therefore, it is recommended to use both methods, detect the device used and detect the device’s resolution for the highest efficiency to avoid false positives.
The following is the code to detect resolution of the device that the user is operating.
function detectMob() {
return ((window.innerWidth <= 800) && (window.innerHeight <= 600));
}
Detect Mobile Browser by Touch Screen
Another approach to check if the target device operated by the user is a mobile device or not is by checking if the user is using a touch-operated device. Since all the mobile phones available on the market today are touch-operated, this method is reliable and efficient.
However, there is one limitation that this method will also include tablets since they are touch-operated as well.
We can use the following code to detect a mobile browser by seeing if the device operates using a touch screen.
var touchDevice = ('ontouchstart' in document.documentElement);
This function can be expanded further to not only include mobile phones and tablets but desktops and laptops supporting touch screens as well.
var touchDevice = ('ontouchstart' in document.documentElement);