How to Detect Browser or Tab Closing Event in JavaScript
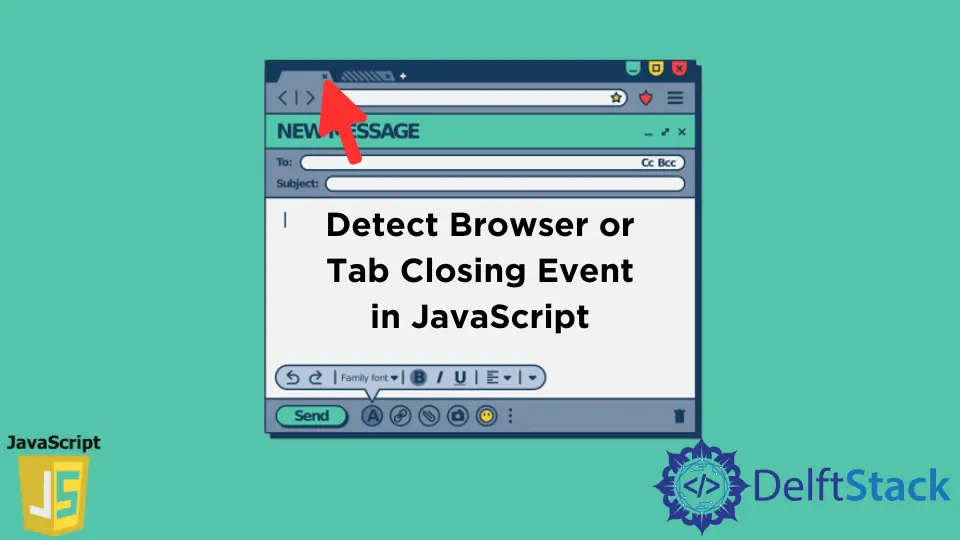
The beforeunload
event is used to detect whether the browser or tab is being closed or the web page is being reloaded.
Use the beforeunload
Event to Detect Browser or Tab Closing Event in JavaScript
The beforeunload
event alerts the users. The addEventListener()
function listens to the event when occurs.
Before unloading the windows & their resources, the beforeunload
event is triggered.
HTML Code (index.html
):
<!DOCTYPE html>
<html>
<head>
<title>
Detect Browser or Tab Closing Event in JavaScript
</title>
<script src = "./script.js"></script>
</head>
<body>
<h1>
Detect Browser or Tab Closing Event in JavaScript
</h1>
<p>
The beforeunload event is fired just
before the closing the tab or browser
window or reloading the page.
In modern web browsers, you may have
to interate with the web
page to get the confirmation dialog.
</p>
<form>
<textarea placeholder = "Write few words here to trigger an
interaction">
</textarea>
</form>
</body>
</html>
JavaScript Code (script.js
):
window.addEventListener('beforeunload', function(e) {
e.preventDefault();
e.returnValue = '';
});
Here, the preventDefault()
is used to show the pop-up for further confirmation. The users have two choices here, either they can navigate to another page or cancel the event and remain on the current page.
Output:
You may have a question about why we have to fill the <textarea>
to get the desired results.
Some web browsers may not decide whether they should present a confirmation dialogue until the users have interacted with the web page.
In this way, we can detect both events, the tab closing and closing the browser window.