How to Get Currently Selected Option in JavaScript
- Understanding the Select Element
- Method 1: Using JavaScript to Get Selected Option
- Method 2: Using the SelectedIndex Property
- Method 3: Using Event Listeners for Dynamic Selection
- Conclusion
- FAQ
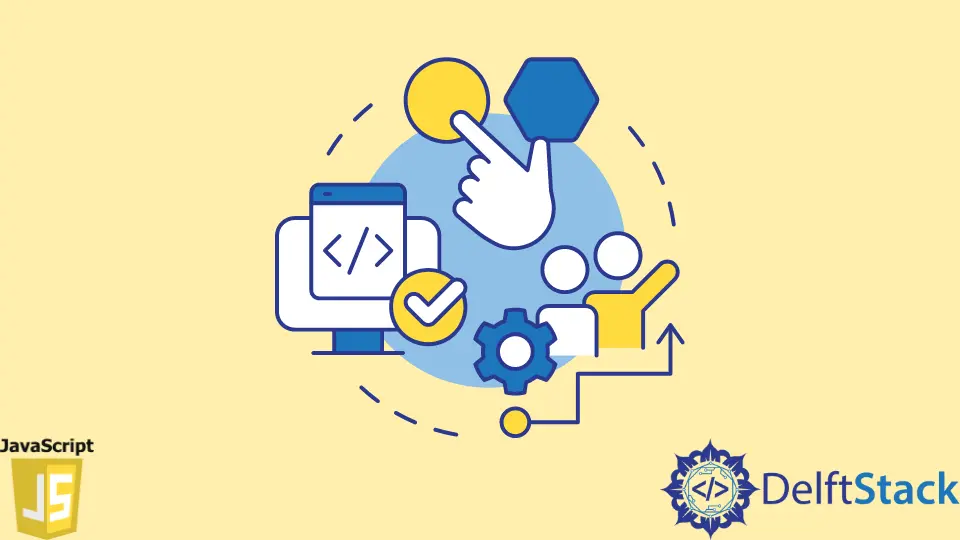
In web development, handling user input is crucial for creating dynamic and interactive applications. One common scenario involves retrieving the currently selected option from a dropdown list, also known as a select element.
In this tutorial, we will explore how to effectively use JavaScript to return the currently selected option from a select list. Whether you’re building a form or a user interface, understanding how to manipulate select elements will enhance your web applications significantly. Let’s dive into the methods you can use to achieve this and see practical examples along the way.
Understanding the Select Element
Before we jump into the code, it’s essential to grasp what a select element is. In HTML, a select element allows users to choose one or more options from a dropdown list. Each option is represented by an <option>
tag nested within the <select>
tag. Here’s a simple example of a select element:
<select id="mySelect">
<option value="option1">Option 1</option>
<option value="option2">Option 2</option>
<option value="option3">Option 3</option>
</select>
In this example, users can choose between three options. The next step is to learn how to retrieve the selected option using JavaScript.
Method 1: Using JavaScript to Get Selected Option
One of the simplest ways to get the currently selected option is by directly accessing the value
property of the select element. This method is straightforward and effective for most use cases. Here’s how you can implement it:
const selectElement = document.getElementById('mySelect');
const selectedOption = selectElement.value;
console.log(selectedOption);
Output:
option1
In this code snippet, we first retrieve the select element using document.getElementById()
. Then, we access the value
property, which returns the value of the currently selected option. Finally, we log the selected option to the console. This method is efficient and works seamlessly for single select elements.
Method 2: Using the SelectedIndex Property
Another effective approach to get the currently selected option is by using the selectedIndex
property. This method is particularly useful if you want to retrieve more information about the selected option, such as its text. Here’s how to do it:
const selectElement = document.getElementById('mySelect');
const selectedIndex = selectElement.selectedIndex;
const selectedOptionText = selectElement.options[selectedIndex].text;
console.log(selectedOptionText);
Output:
Option 1
In this example, we first get the index of the currently selected option using selectedIndex
. Then, we access the corresponding option from the options
collection of the select element. By using text
, we can retrieve the visible text of the selected option. This method provides more flexibility, especially when you need to display user-friendly information.
Method 3: Using Event Listeners for Dynamic Selection
If you want to respond to user interactions dynamically, using event listeners is an excellent approach. You can set up an event listener that triggers whenever the user changes the selected option. Here’s how to implement this:
const selectElement = document.getElementById('mySelect');
selectElement.addEventListener('change', function() {
const selectedOption = this.value;
console.log(selectedOption);
});
Output:
option2
In this code, we add an event listener to the select element that listens for the change
event. When the user selects a different option, the function is triggered, and we log the newly selected option to the console. This method is particularly useful for interactive applications where the UI needs to update based on user selection.
Conclusion
In this tutorial, we explored various methods for retrieving the currently selected option from a select list using JavaScript. From accessing the value
property to utilizing event listeners, each approach has its unique advantages depending on the requirements of your application. Understanding how to manipulate select elements will undoubtedly enhance your web development skills and improve user experience. As you continue to build interactive web applications, mastering these techniques will be invaluable.
FAQ
-
How can I get the selected option in a multi-select dropdown?
You can use theselectedOptions
property to retrieve all selected options in a multi-select dropdown. -
Can I get the selected option without using an event listener?
Yes, you can directly access the select element and use thevalue
orselectedIndex
properties to get the selected option.
-
Is it possible to change the selected option using JavaScript?
Absolutely! You can set thevalue
property of the select element to change the selected option programmatically. -
What if I want to get the selected option using jQuery?
You can use$('#mySelect').val()
in jQuery to retrieve the selected option easily. -
How do I handle form submissions with selected options?
When the form is submitted, the selected option will automatically be included in the form data, which you can then process on the server side.