How to Create Div Element in JavaScript
- Understanding the Basics of the Div Element
- Creating a Div Element Using JavaScript
- Styling the Div Element
- Adding Event Listeners to the Div
- Conclusion
- FAQ
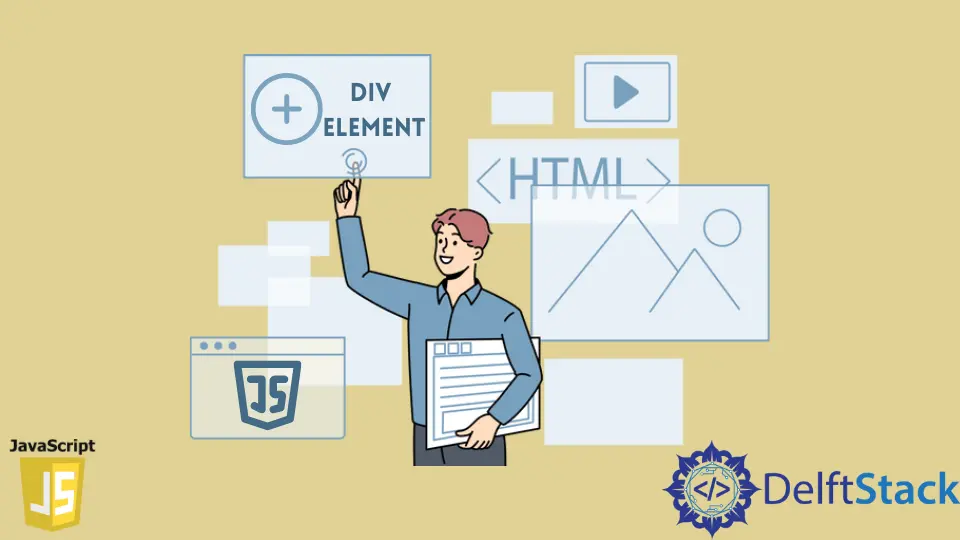
Creating and styling a div element in JavaScript is a fundamental skill for web developers. Whether you’re building a simple webpage or a complex application, knowing how to manipulate the Document Object Model (DOM) is crucial.
This tutorial will guide you through the process of creating a div element using JavaScript, along with some styling techniques to make your div stand out. By the end of this article, you’ll have a solid understanding of how to dynamically create and style div elements, enhancing your web development toolkit.
Understanding the Basics of the Div Element
The div element is a block-level container in HTML that is commonly used to group other elements together. It serves as a versatile tool for layout design, allowing developers to apply CSS styles and JavaScript functionality. Understanding how to create and manipulate div elements is essential for any web developer.
In this section, we will explore how to create a div element using JavaScript, including how to set its attributes and styles. This knowledge will empower you to build dynamic and interactive web pages.
Creating a Div Element Using JavaScript
To create a div element in JavaScript, you can use the document.createElement
method. This method allows you to generate a new HTML element dynamically. Here’s how you can do it:
const newDiv = document.createElement('div');
newDiv.style.width = '60px';
newDiv.style.height = '20px';
newDiv.style.background = 'green';
newDiv.style.color = 'white';
newDiv.innerHTML = 'Upwork';
document.body.appendChild(newDiv);
Output:
In this code snippet, we first create a new div element using document.createElement('div')
. We then set its inner text to provide some content. Next, we apply some CSS styles directly through JavaScript, including a background color, padding, and a border. Finally, we append the new div to the body of the document using document.body.appendChild(newDiv)
. This method effectively adds the newly created div to the webpage.
Styling the Div Element
Once you have created a div element, you might want to style it further to enhance its appearance. You can achieve this by modifying its CSS properties directly in JavaScript. Here’s how:
newDiv.style.color = "white";
newDiv.style.fontSize = "20px";
newDiv.style.textAlign = "center";
Output:
In this example, we are changing the text color to white, increasing the font size, and centering the text within the div. These styles can significantly improve the visual appeal of your div. Adjusting styles dynamically like this can make your web applications more interactive and user-friendly.
Adding Event Listeners to the Div
To make your div element interactive, you can add event listeners. This allows you to respond to user actions, such as clicks or mouse movements. Here’s how you can add a click event to your div:
newDiv.addEventListener('click', () => {
alert('Div was clicked!');
});
Output:
By using the addEventListener
method, we can attach a click event to the new div. When the user clicks on the div, an alert box will pop up displaying the message “Div was clicked!” This interactivity can enhance user engagement and create a more dynamic experience on your webpage.
Conclusion
Creating and styling div elements in JavaScript is a fundamental skill that every web developer should master. By using methods like document.createElement
, applying CSS styles directly, and adding event listeners, you can significantly enhance the interactivity and aesthetics of your web applications. As you continue to practice these techniques, you’ll find that they open up a world of possibilities for creating dynamic, user-friendly websites. Remember, the key to mastering JavaScript is consistent practice and experimentation.
FAQ
-
How do I create multiple div elements using JavaScript?
You can use a loop to create multiple div elements. For example, a for loop can be used to create several divs and append them to the body. -
Can I style div elements using CSS classes in JavaScript?
Yes, you can assign a class to the div usingnewDiv.className = 'your-class-name';
and define styles in your CSS file. -
What other attributes can I set for a div element?
You can set various attributes likeid
,class
, anddata-*
attributes usingnewDiv.setAttribute('attributeName', 'value');
. -
Is it possible to remove a div element using JavaScript?
Yes, you can remove a div by selecting it and using theremove()
method, or by usingparentElement.removeChild()
.
- Can I create nested div elements in JavaScript?
Absolutely! You can create a div and then create another div inside it, appending the inner div to the parent div before adding the parent to the document.
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedIn