How to Convert UTC to Local Time in JavaScript
Siddharth Swami
Feb 02, 2024
-
Use the
tostring()
Function to Convert UTC to Local Time in JavaScript -
Use the
Date()
Method to Convert UTC to Local Time in JavaScript
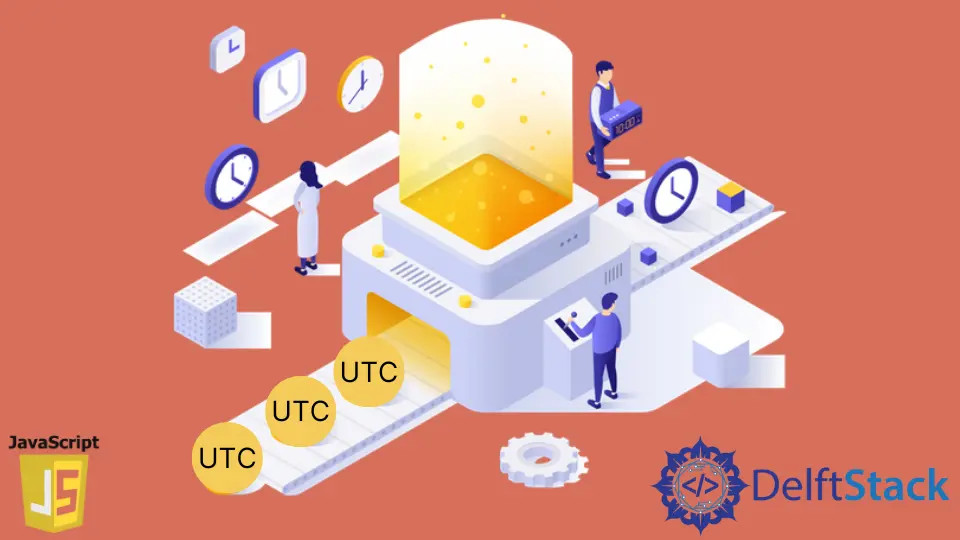
This tutorial demonstrates how to convert UTC to local time in JavaScript.
Use the tostring()
Function to Convert UTC to Local Time in JavaScript
JavaScript allows us to convert the UTC to local time with the help of a method known as toString()
.
For example,
var dt = new Date('7/24/2021 2:11:55 PM UTC');
console.log(dt.toString())
Output:
"Sat Jul 24 2021 16:11:55 GMT+0200 (Central European Summer Time)"
In the above example, the final date and time are based on the local time zone. The variable dt
consists of the user-specified date-time in UTC in a Date
object.
Use the Date()
Method to Convert UTC to Local Time in JavaScript
We create objects to store date using the Date()
method in JavaScript. When we store the date in ISO 8601 format, the server returns the date and time based on the local time zone.
For example,
var dt = new Date(
'2021-07-24T20:37:26.007' +
'Z');
console.log(dt.toLocaleString());
Output:
"7/24/2021, 10:37:26 PM"