How to Convert JSON to Object in JavaScript
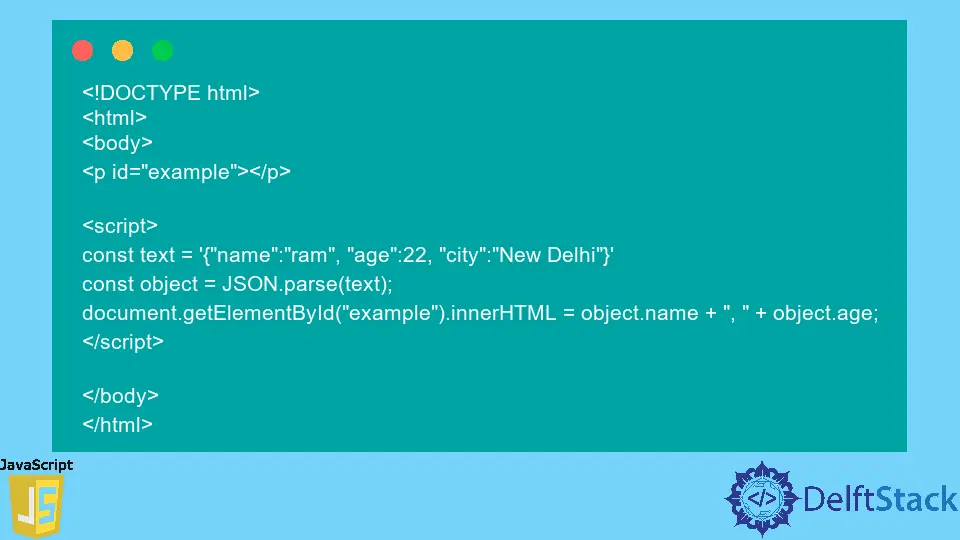
JSON is light and text-based language for storing and transferring data. JSON represents two structured types that are objects and arrays. It is based on JavaScript objects and stands for JavaScript Object Notation. JSON is primarily used in the exchange of data to/from the web.
This tutorial demonstrates how safely turn a JSON string into an object.
Data is received from the server in the form of a string, either numeric value or date. So we must change data into an object to execute the file. We have a function known as JSON.parse()
in JavaScript used to parse the data that becomes a JavaScript object. The function parses the complete text provided by the server and then changes it to an object.
The following code demonstrates the use of JSON.parse()
function.
<!DOCTYPE html>
<html>
<body>
<p id="example"></p>
<script>
const text = '{"name":"ram", "age":22, "city":"New Delhi"}'
const object = JSON.parse(text);
document.getElementById("example").innerHTML = object.name + ", " + object.age;
</script>
</body>
</html>
Output:
ram, 22
In the above example, we have a JSON text which consists of a name and the city where a person lives, which is then parsed by making an object for it. Then using the getElementById()
function, we can get the required values.
Another way to use the JSON.parse()
is by using the let()
function in JavaScript. Here we can directly parse the text without first assigning it to a variable and then parsing it.
See the following code.
<!DOCTYPE html>
<html>
<body>
<p id="example"></p>
<script>
let json_Object = JSON.parse('{"name":"ram", "age":22, "city":"New Delhi"}');
document.getElementById("example").innerHTML = json_Object.name + ", " + json_Object.age;
</script>
</body>
</html>
Output:
ram, 22
In JSON, date objects are not allowed. So we have to write it as a string in JSON and then convert it back into a date object using the Date()
function.
For example,
<!DOCTYPE html>
<html>
<body>
<p id="example"></p>
<script>
const text1 = '{"name":"ram", "birth":"2000-03-05", "city":"New Delhi"}';
const object = JSON.parse(text1);
object.birth = new Date(object.birth);
document.getElementById("example").innerHTML = object.birth;
</script>
</body>
</html>
Output:
Sun Mar 05 2000 05:30:00 GMT+0530(India Standard Time)
Related Article - JavaScript JSON
- How to Format JSON in JavaScript
- How to Generate Formatted and Easy-To-Read JSON in JavaScript
- How to Convert XML to JSON in JavaScript
- How to Convert CSV to JSON in JavaScript
- How to POST a JSON Object Using Fetch API in JavaScript
- How to Convert JSON to XML in JavaScript