How to Convert Integer to String in JavaScript
- Method 1: Using the String Constructor
-
Method 2: Using the
toString()
Method - Method 3: Using Template Literals
- Method 4: Using String Concatenation
- Method 5: Using JSON.stringify()
- Conclusion
- FAQ
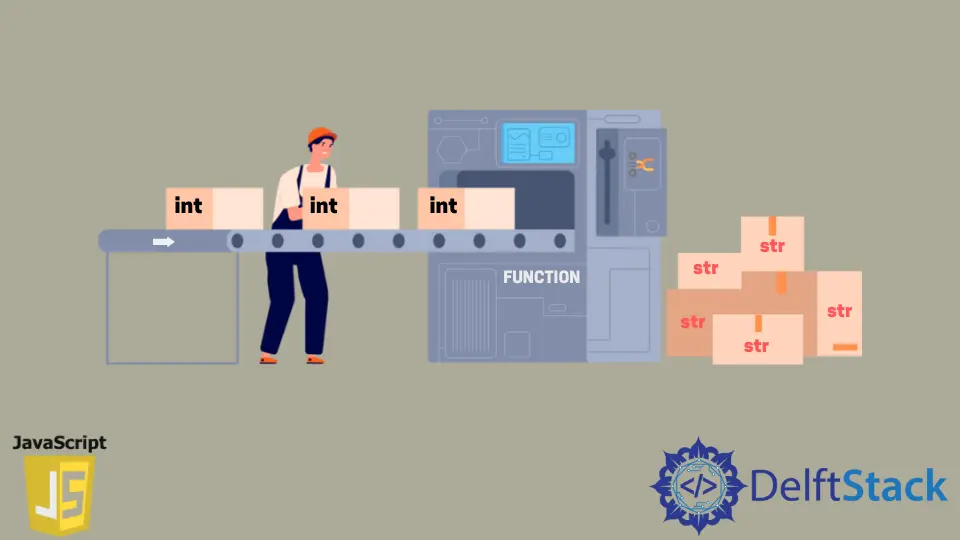
Converting an integer to a string in JavaScript is a common task that developers encounter frequently. Whether you are formatting numbers for display, concatenating values, or preparing data for storage, understanding how to perform this conversion is essential.
In this tutorial, we will explore various methods to convert integers to strings in JavaScript, providing clear examples and explanations. By the end, you will have a solid grasp of how to effortlessly handle this conversion in your JavaScript projects.
Method 1: Using the String Constructor
One of the simplest ways to convert an integer to a string in JavaScript is by using the String()
constructor. This method is straightforward and effective, making it a popular choice among developers.
Here’s how you can do it:
let number = 123;
let stringValue = String(number);
console.log(stringValue);
Output:
123
In this example, we define a variable number
with an integer value of 123. By passing this variable to the String()
constructor, we convert it into a string and store the result in stringValue
. The console.log()
function then displays the string value in the console. This method is particularly useful when you want to ensure that the conversion is done safely, as it handles non-string inputs gracefully.
Method 2: Using the toString()
Method
Another effective method to convert an integer to a string in JavaScript is by utilizing the toString()
method. This method is built into the Number object and can be called directly on any number.
Here’s an example:
let number = 456;
let stringValue = number.toString();
console.log(stringValue);
Output:
456
In this snippet, we have an integer stored in the variable number
. By calling the toString()
method on this variable, we convert it into a string and assign it to stringValue
. This method is particularly convenient as it allows for direct conversion without the need for any additional functions. It’s worth noting that toString()
can also accept a radix parameter, allowing you to convert numbers to different bases if needed.
Method 3: Using Template Literals
Template literals are a powerful feature in JavaScript that allows for easier string interpolation. They can also be used to convert integers to strings seamlessly.
Here’s how you can use template literals for this purpose:
let number = 789;
let stringValue = `${number}`;
console.log(stringValue);
Output:
789
In this example, we define a variable number
with an integer value of 789. By enclosing the variable in backticks and using the ${}
syntax, we create a template literal that automatically converts the integer to a string. This method is particularly useful when you want to include variables within strings, as it enhances readability and conciseness.
Method 4: Using String Concatenation
String concatenation is another simple yet effective way to convert an integer to a string in JavaScript. By concatenating an integer with an empty string, you can force the conversion.
Here’s a quick example:
let number = 101112;
let stringValue = number + '';
console.log(stringValue);
Output:
101112
In this snippet, we take the integer number
and concatenate it with an empty string. This operation coerces the integer into a string, and the result is stored in stringValue
. This method is quite handy and can be used in various contexts, especially when you need a quick conversion without additional function calls.
Method 5: Using JSON.stringify()
If you are dealing with more complex data structures or want to ensure that your conversion handles various data types correctly, JSON.stringify()
is an excellent option. While primarily used for converting objects to JSON strings, it can also convert numbers to strings.
Here’s how it works:
let number = 2023;
let stringValue = JSON.stringify(number);
console.log(stringValue);
Output:
2023
In this example, we use JSON.stringify()
to convert the integer number
into a string. This method is particularly useful when you are working with objects or arrays, as it ensures that all data types are converted correctly. While it may seem a bit unconventional for simple integer-to-string conversions, it can be a lifesaver in more complex scenarios.
Conclusion
Converting integers to strings in JavaScript is a fundamental skill that every developer should master. In this tutorial, we explored several methods, including the String constructor, toString()
method, template literals, string concatenation, and JSON.stringify()
. Each method has its own advantages, and understanding when to use each one can enhance your coding efficiency and effectiveness. Whether you are formatting data for user interfaces or processing inputs, knowing how to convert integers to strings will ensure that your JavaScript applications run smoothly.
FAQ
-
What is the easiest way to convert an integer to a string in JavaScript?
The easiest way is to use the String constructor, like this:String(number)
. -
Can I use the
toString()
method on non-integer values?
Yes, thetoString()
method can be used on any number, including floats and negative numbers. -
Are there any performance differences between these methods?
Generally, the performance differences are negligible for small numbers, but usingtoString()
or the String constructor is often the most efficient. -
Can I convert multiple integers to strings at once?
You can use an array and map over it to convert multiple integers to strings using any of the methods discussed. -
What should I do if my number is very large?
JavaScript can handle large integers, but if you encounter issues, consider using BigInt for larger values.