Constants in JavaScript
- What are Constants in JavaScript?
- When to Use Constants
- Benefits of Using Constants
- Common Mistakes with Constants
- Best Practices for Using Constants
- Conclusion
- FAQ
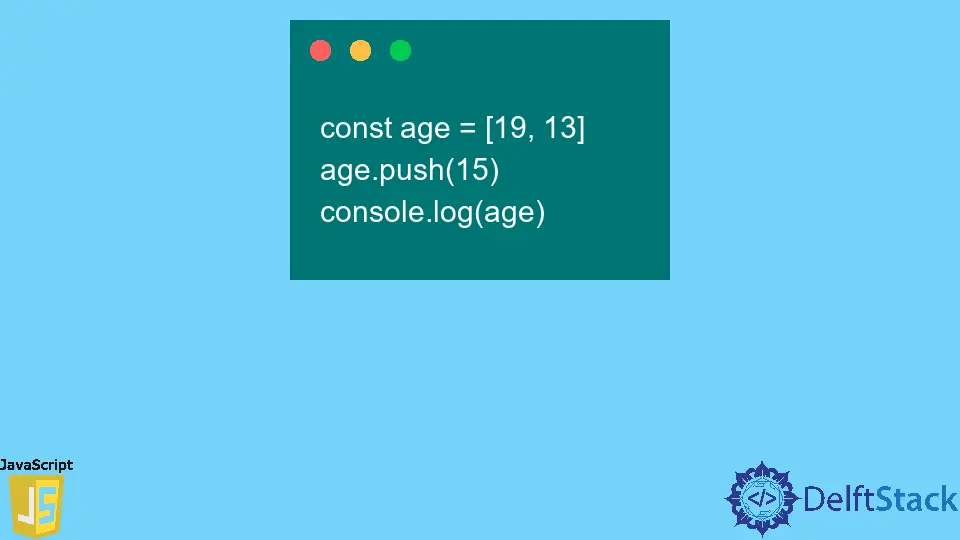
JavaScript is a versatile programming language that powers the web. One of its key features is the ability to define constants, which are values that cannot be reassigned after their initial definition. Understanding when and how to use constants can greatly enhance your code’s readability and maintainability.
In this tutorial, we will explore the significance of constants in JavaScript, how to declare them, and the best practices for using them effectively. Whether you are a beginner or an experienced developer, mastering constants is essential for writing cleaner and more efficient JavaScript code.
What are Constants in JavaScript?
In JavaScript, constants are defined using the const
keyword. A constant is a block-scoped variable whose value cannot be changed through reassignment. This means that once you assign a value to a constant, you cannot change it later in your code. This feature is particularly useful for values that should remain constant throughout the execution of your program, such as configuration settings or fixed values.
Here is a simple example of declaring a constant:
const pi = 3.14;
In this case, pi
is a constant that holds the value of pi. Attempting to change its value later in the code will result in an error.
Output:
TypeError: Assignment to constant variable.
Using constants helps prevent accidental changes to values that should remain static, making your code more predictable and easier to debug.
When to Use Constants
Constants should be used in situations where the value is not intended to change. This can include:
- Configuration values, such as API keys or URLs.
- Mathematical constants like pi or Euler’s number.
- Fixed strings that are used throughout your application.
By using constants, you can signal to other developers (and yourself) that these values are not meant to be modified. This improves code clarity and reduces the likelihood of bugs.
Here’s an example of using constants in a configuration setting:
const API_URL = "https://api.example.com/v1";
In this example, API_URL
is a constant that holds the base URL for API requests. Since this value should not change during the execution of the program, declaring it as a constant is the best choice.
Using constants for configuration values helps maintain consistency and reduces errors when accessing these values throughout your code.
Benefits of Using Constants
Using constants in your JavaScript code brings several benefits:
-
Predictability
: Since constants cannot be reassigned, you can trust that their values will remain the same throughout your program. This predictability makes debugging easier. -
Readability
: Constants can serve as meaningful names for values, making your code more understandable. For instance, usingconst MAX_USERS = 100;
is clearer than using the literal number100
throughout your code. -
Performance
: While the performance benefits may be negligible, using constants can help the JavaScript engine optimize your code better since it knows certain values will not change.
Here’s an example demonstrating the readability aspect:
const MAX_RETRIES = 5;
for (let i = 0; i < MAX_RETRIES; i++) {
// Attempt to connect to the server
}
In this example, MAX_RETRIES
clearly indicates the maximum number of attempts to connect to the server, improving code clarity.
By using constants, you enhance the overall quality of your code, making it easier for others to read and maintain.
Common Mistakes with Constants
While constants are a powerful feature in JavaScript, there are common pitfalls to avoid. One common mistake is confusing constants with immutable objects. While you cannot reassign a constant, the contents of an object or array defined with const
can still be modified.
Here’s an example:
const myArray = [1, 2, 3];
myArray.push(4);
In this case, even though myArray
is declared as a constant, you can still modify its contents by adding new elements.
Output:
myArray is now [1, 2, 3, 4]
To prevent confusion, it’s essential to understand that const
only protects the variable binding, not the value itself. This distinction is crucial when working with complex data structures.
Best Practices for Using Constants
To make the most out of constants in your JavaScript code, consider these best practices:
-
Use Uppercase for Constant Names
: By convention, constants are often written in uppercase letters with underscores separating words. This helps differentiate them from regular variables. -
Group Related Constants
: If you have multiple related constants, consider grouping them together in an object. This approach keeps your code organized and makes it easier to manage. -
Limit Scope
: Use block scope for constants whenever possible. This practice prevents unintended access and modifications from other parts of your code.
Here’s an example of grouping constants:
const CONFIG = {
API_URL: "https://api.example.com/v1",
MAX_USERS: 100,
TIMEOUT: 5000
};
In this example, the CONFIG
object groups related constants together, enhancing organization and readability.
By following these best practices, you can ensure that your use of constants is effective and contributes to clean, maintainable code.
Conclusion
Constants are a fundamental aspect of JavaScript that can significantly improve your coding practices. By using the const
keyword, you can create variables that remain unchanged throughout your program, enhancing readability, predictability, and maintainability. Whether you are working with configuration values, mathematical constants, or fixed strings, understanding when and how to use constants is essential for any JavaScript developer. By following best practices and avoiding common pitfalls, you can leverage the power of constants to write cleaner and more efficient code.
FAQ
- what is the difference between const and let in JavaScript?
const defines a constant variable that cannot be reassigned, while let defines a block-scoped variable that can be reassigned.
-
can I change the value of an object defined with const?
No, you cannot reassign the object itself, but you can modify its properties. -
when should I use constants in my code?
Use constants for values that should remain unchanged, such as configuration settings or fixed values. -
are there any performance benefits to using constants?
While performance gains may be minimal, using constants can help the JavaScript engine optimize your code. -
what naming convention should I follow for constants?
It is common to use uppercase letters with underscores separating words for constant names.