How to Compare Strings in JavaScript
-
Compare Strings With the
localeCompare()
Method in JavaScript - Compare Two Strings With User-Defined Function in JavaScript
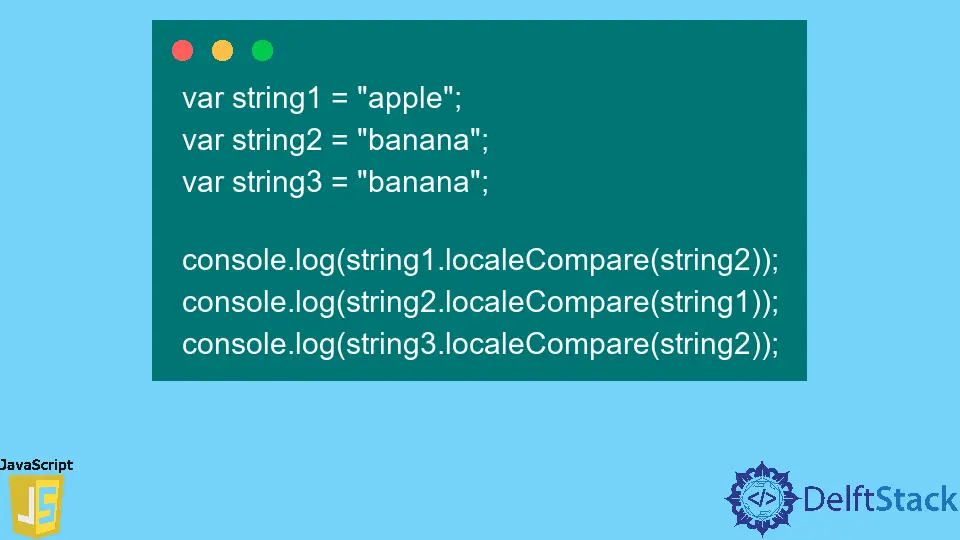
If we want to compare a string in JavaScript, the corresponding task is done by the method localeCompare()
. You can also define a function that can terminate to preferable cases.
Here we will disclose about two ways of comparing two strings. One is by the localeCompare()
method, and the other is by the user-defined
function.
Compare Strings With the localeCompare()
Method in JavaScript
In this case, we will compare string1
with string2
. The driven result gives three return types, -1
, 0
, 1
.
- If
string1
is after (greater alphabetically, or chronologically than)string2
, the return value will be1
. - If
string1
is before (smaller than)string2
, the method will return-1
. - If they are the same, the return value will be
0
.
The following example will focus on the localeCompare()
method to compare strings.
Code Snippet:
var string1 = 'apple';
var string2 = 'banana';
var string3 = 'banana';
console.log(string1.localeCompare(string2));
console.log(string2.localeCompare(string1));
console.log(string3.localeCompare(string2));
Output:
-1
1
0
Compare Two Strings With User-Defined Function in JavaScript
Here, we have initialized a function compare
that takes two comparable strings as the argument. There is also a conditional segment to define the return cases. As a result, we will get return cases according to the function drive.
Code Snippet:
var string1 = 'apple';
var string2 = 'banana';
var string3 = 'banana';
function compare(string1, string2) {
if (string1 < string2) {
return -1;
} else if (string1 > string2) {
return 1;
} else {
return 0;
}
}
console.log(compare(string1, string2));
console.log(compare(string2, string1));
console.log(compare(string2, string3));
Output:
-1
1
0