How to Colon in JavaScript
- Colon in the Ternary Operation in JavaScript
- Colon in JavaScript for Object Literal Structure
-
Use Colon in a
Switch-Case
Statement - Set Labels With Colon in JavaScript
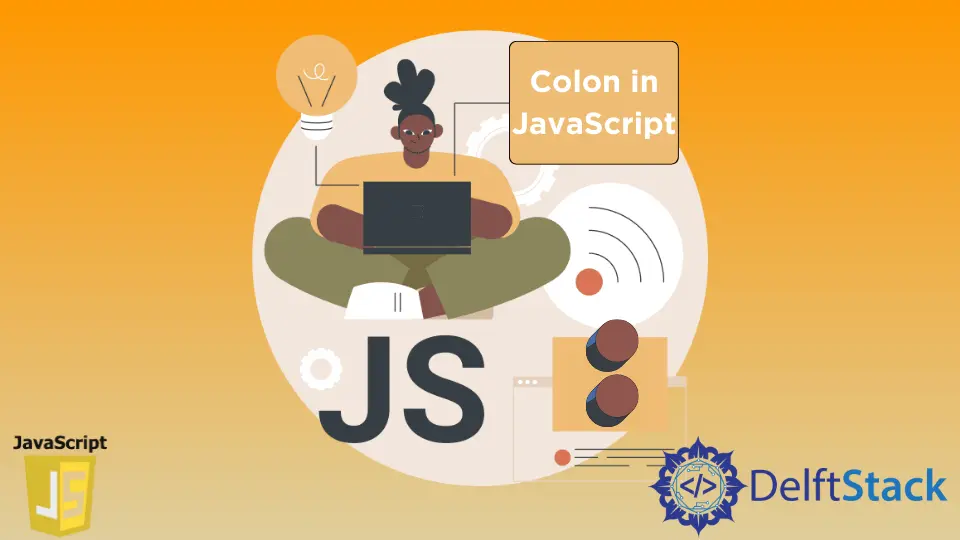
Colon (:)
in JavaScript can be used in multiple cases. We will describe the colon convention in the ternary operator, JavaScript object literal structure, switch-case
statements, and go-to
statements or setting labels.
Colon in the Ternary Operation in JavaScript
The conditional operation in JavaScript is also doable in inline structure, and this specification of the if-else
statement looks more neat and clear.
Firstly, a condition is applied upon which a statement will execute, and if that fails, the next statement will be executed. Here the dissection symbol between the statements is the colon.
var p = 42;
var q = 7;
console.log(p > q ? 'It\'s ' + p : 'Oops it\'s ' + q);
Output:
Colon in JavaScript for Object Literal Structure
In the case of object literal structure in JavaScript, the key-value pairs are set with a colon. The left side of the colon is the keys
, and the right side of the colon is for the value
.
var object = {hair: 'long', color: 'black', age: 42}
console.log(object.color + object.age);
Output:
Use Colon in a Switch-Case
Statement
A switch-case
statement has multiple cases for drawing out the apt solution.
While initializing the cases, we separate them with the code-block with a colon. Even the default statement in the switch-case
is directed with a colon.
var day;
switch (new Date().getDay()) {
case 6:
day = 'It\'s Saturday';
break;
case 0:
day = 'It\'s Sunday';
break;
default:
day = 'Oh it\'s not holiday.'
}
console.log(day);
Output:
Set Labels With Colon in JavaScript
This coding practice is not very much appreciated in most cases but can aid in many problems. After labeling a certain code block, we use a colon right after the label.
var x = 5;
var y = 42;
var z = 0;
sum: while (x < y) {
z = x++;
if (x >= y) {
break sum;
}
}
console.log(z);
Output: