How to Collapse or Expand a DIV in JavaScript
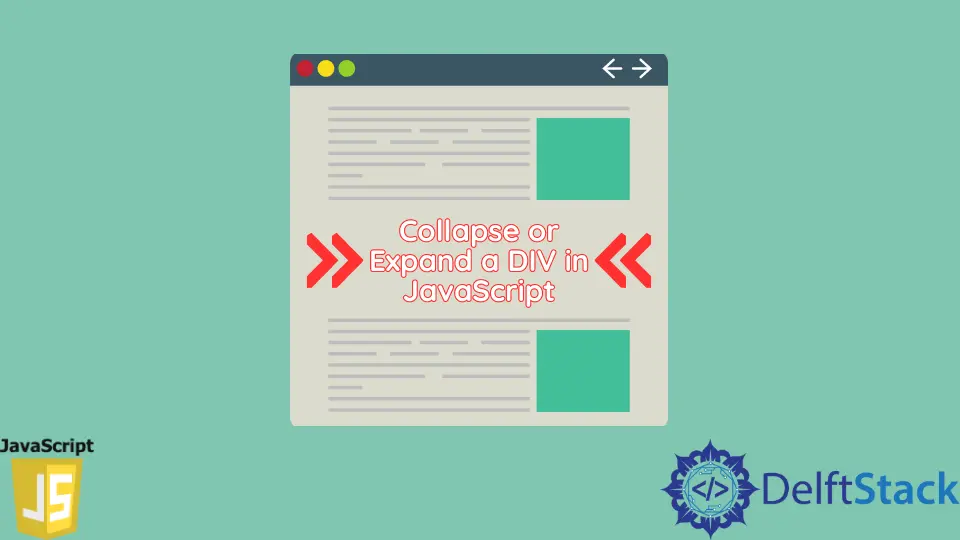
jQuery
is a javascript library that is light and very fast. It simplifies the use of JavaScript.
A div
tag is a division tag. It is used to define a section in an HTML document. It also acts as a container for other HTML elements.
In this tutorial, we will collapse or expand a div
using jQuery
.
Check the example given below.
<fieldset class="click" >
<legend class="buttonMain" style="cursor: pointer">Expand</legend>
<div class="content" style="display:none">
<p>Lorem ipsum...</p>
</div>
The above HTML code contains some elements, including the div
tag. We are manipulating the div
using jQuery
to make it collapsible.
In jQuery
, the show()
and hide()
method are used to show or hide any element in HTML.
We can use these functions to collapse or expand the div
tag as shown below.
$('.buttonMain').click(function() {
if ($(this).text() == 'Expand') {
$('.content').show();
$(this).text('Collapse');
} else {
$('.content').hide();
$(this).text('Expand');
}
});
We can also specify the speed for hiding and showing elements using the speed
parameter in these functions. We can determine its value as slow
, fast
, or speed in milliseconds.