How to Clear Console Using JavaScript
- Understanding the Console in JavaScript
- Method 1: Using console.clear()
- Method 2: Using Keyboard Shortcuts
- Method 3: Refreshing the Page
- Conclusion
- FAQ
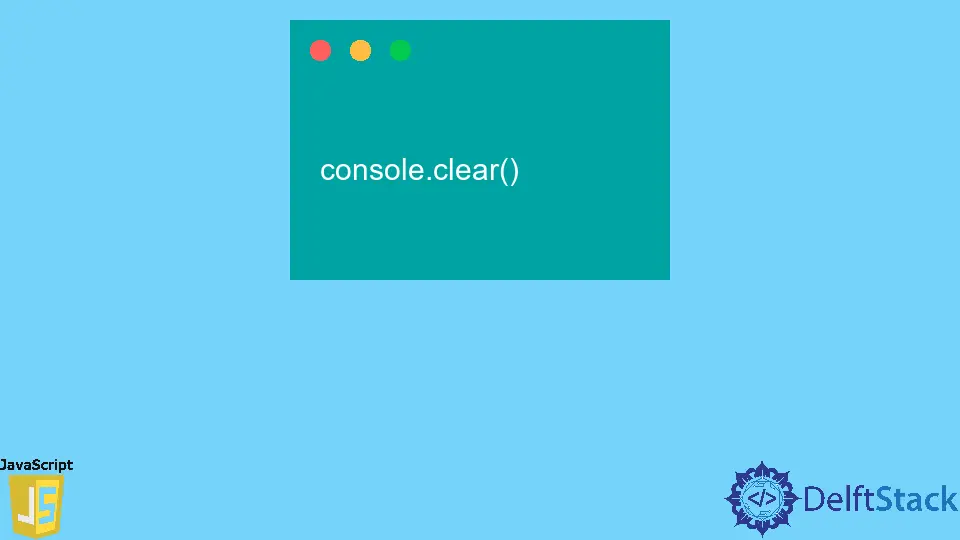
In today’s post, we’ll learn how to clear console using JavaScript. Whether you are debugging your code or simply want to keep your console output tidy, knowing how to clear the console is a fundamental skill for any developer. The console is a powerful tool that allows you to log messages, inspect variables, and track the flow of your application. However, as you work, it can quickly become cluttered with unnecessary information.
In this article, we will explore various methods to clear the console in JavaScript, ensuring that you can maintain a clean workspace and focus on what matters most: writing great code.
Understanding the Console in JavaScript
Before we delve into the methods for clearing the console, it’s essential to understand what the console is and why it matters. The console is part of the browser’s developer tools, and it provides a way to interact with your web application in real-time. You can log errors, warnings, and informational messages, making it easier to debug your code. However, as you log more messages, the console can become overwhelming. Clearing it helps you focus on the most recent outputs, making it easier to identify problems and track your application’s behavior.
Method 1: Using console.clear()
One of the simplest ways to clear the console in JavaScript is by using the console.clear()
method. This built-in function will remove all messages from the console, providing you with a clean slate to work with. Here’s how you can implement it:
console.log("This is a message before clearing the console.");
console.clear();
console.log("This is a message after clearing the console.");
Output:
This is a message before clearing the console.
When you run this code, the first message will appear in the console, but after calling console.clear()
, the console will be cleared. The second message will then be logged. This method is straightforward and works in most modern browsers, making it a go-to option for many developers. However, keep in mind that the use of console.clear()
may vary depending on the browser’s settings and user preferences, as some browsers allow users to disable this feature.
Method 2: Using Keyboard Shortcuts
If you prefer a quicker way to clear the console without writing any code, you can use keyboard shortcuts. Most browsers offer built-in shortcuts that allow you to clear the console instantly. Here are the common shortcuts for various browsers:
- Google Chrome: Press Ctrl+L (Windows/Linux) or Command+K (Mac).
- Firefox: Press Ctrl+L (Windows/Linux) or Command+K (Mac).
- Safari: Press Command+K (Mac).
Using these shortcuts is a convenient way to maintain a clean console without interrupting your coding flow. While this method doesn’t require any JavaScript knowledge, it’s a handy tool to have in your developer toolkit. Keep in mind that these shortcuts may vary slightly depending on your operating system and browser version, so it’s always good to double-check the documentation for your specific setup.
Method 3: Refreshing the Page
Another way to clear the console is by refreshing the page. This method is more of a workaround than a direct method for clearing the console, but it effectively resets the console output. Here’s how you can do it:
console.log("This message will be cleared after refreshing the page.");
// Refresh the page to clear the console
Output:
This message will be cleared after refreshing the page.
When you refresh the page, all console messages will disappear, and you’ll start with a clean slate. This method is particularly useful when you want to reset the entire state of your application, not just the console. However, be aware that refreshing the page will also reset any JavaScript variables and application state, so use this method with caution if you’re working on a complex application.
Conclusion
In conclusion, knowing how to clear the console using JavaScript is an essential skill for developers. Whether you choose to use console.clear()
, keyboard shortcuts, or simply refresh the page, each method has its advantages depending on your workflow. Keeping your console tidy not only enhances your debugging experience but also allows you to focus on writing better code. As you continue to develop your skills in JavaScript, mastering these simple techniques will undoubtedly improve your efficiency and effectiveness as a programmer.
FAQ
-
How often should I clear the console?
Clearing the console is a personal preference, but it’s a good idea to do it regularly, especially when you’re debugging or testing new code. -
Does clearing the console affect my application?
No, clearing the console only removes the logged messages and does not impact your application’s functionality or state. -
Can I customize the console output?
Yes, you can use various console methods likeconsole.log()
,console.warn()
, andconsole.error()
to customize how messages are displayed in the console. -
Is
console.clear()
supported in all browsers?
Most modern browsers supportconsole.clear()
, but its behavior may vary based on user settings. -
What happens to messages after I clear the console?
Once you clear the console, all previous messages are removed and cannot be retrieved.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn