How to Validate Checkbox in JavaScript
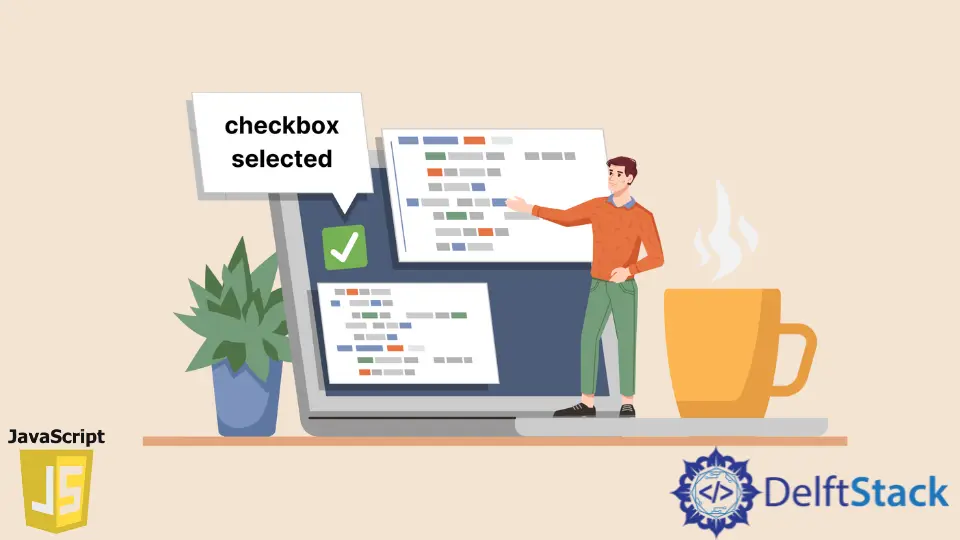
In this article, we will introduce checkbox validation in JavaScript using the checked
property of the input element.
Use the checked
Property to Validate the Checkbox in JavaScript
The input element in JavaScript is used to take input from the user. This can be used inside forms as well.
It provides us with a checked
property to validate the checkbox in JavaScript. The checked
property will return true
if the user checks the checkbox; else, it will return false
.
Below we have an HTML document. We have a label and a button inside the body element.
Using the label
element, we can provide a label to the checkbox. The input
element is wrapped around the label
element.
To make this input a checkbox, we have to set its type to checkbox
, and we also have to provide the input with an ID of checkbox
to access this element inside JavaScript.
<!DOCTYPE html>
<html>
<head>
<body>
<label for="Select Checkbox">
<input type="checkbox" id="checkbox"> Accept
</label>
<button id="submit">Submit</button>
<script>
const checkbox = document.getElementById('checkbox');
const btn = document.getElementById('submit');
btn.onclick = () => {
if(!checkbox.checked)
console.log("Checkbox selected: ", checkbox.checked);
else
console.log("Checkbox selected: ", checkbox.checked);
};
</script>
</body>
</html>
Output:
Here, we first stored the reference of the checkbox
element, which we have defined inside our HTML using DOM API’s getElementById()
method. Similarly, we did this for the submit button as well.
Now that we have the checkbox and the submit button stored inside the checkbox
and btn
variables, we use them to perform validation. Here, we set an onclick()
element on the btn
, which, on the press, will execute a function.
Inside this function, we used a condition statement and checked if the checkbox.checked
is true or not.
Notice that we have used an exclamation mark !
inside the if
block. This means that if the user does not check the checkbox, checkbox.checked
will return false, and !false
will become true
.
Since the user has not selected the checkbox, we entered inside the if
block and then printed Checkbox selected: false
.
Otherwise, if the user clicks the checkbox, we will go inside the else
block, and the message printed on the console will be Checkbox selected: true
.
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn