How to Check if All Values in Array Are True in JavaScript
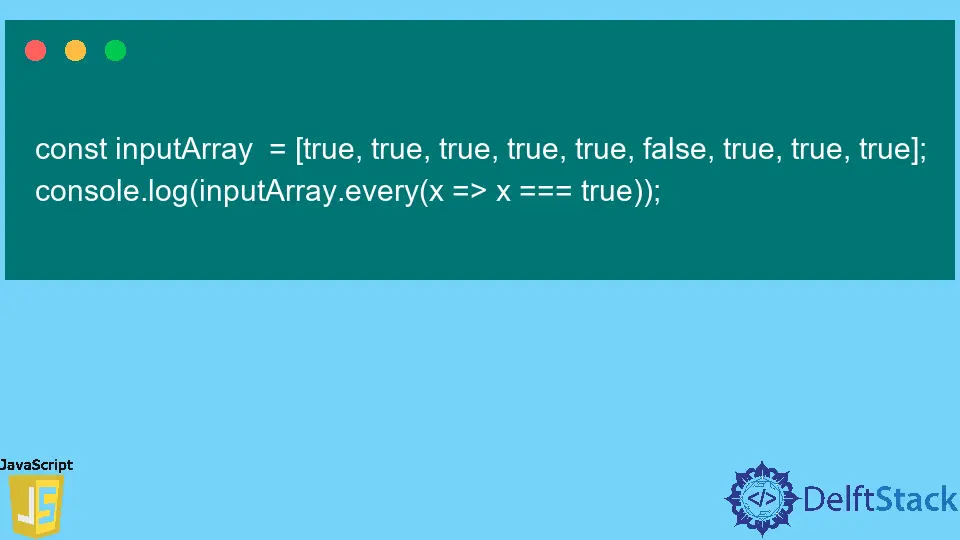
In today’s post, we’ll learn how to check if all values in an array are true or not in JavaScript.
Use the every()
Method to Check if All Values in Array Are True in JavaScript
The every()
method is a built-in method provided by JavaScript that examines whether all elements inside the array pass the condition/criteria carried out through the provided function. Based on the function, it returns either a true
or false
boolean value.
Syntax:
every((element, index) => {/* Logic goes here */})
The first parameter is the current element
processed in the array. The index
parameter is the current element’s index processed in the array.
The every()
method no longer mutates the array on which it’s referred to as. Calling the every()
method on an empty array will return true
for any condition.
This method returns true
if the callbackFn
function returns a truthy value for every array element; otherwise, it returns false
. The falsy values in JavaScript are false
, undefined
, null
, 0
, NaN
(Not a Number) and empty string.
For each element present in the array, the every()
method runs the provided callbackFn
function once until one falsy/incorrect value is found. If such an element is found, the every()
method returns false
immediately, and the remaining elements are skipped.
If callbackFn
returns true
for all elements present in an array, they all return true
. callbackFn
is only called for array indexes that have values assigned.
It is not called for dropped indexes or has not been assigned values.
You can get more information about the every()
method in the documentation for every
.
Let’s understand it with the following example:
const inputArray = [true, true, true, true, true, false, true, true, true];
console.log(inputArray.every(x => x === true));
We have defined an input array with 8 true values and one false value within the above example. When the every()
method encounters a false
value on the fifth index, it will stop the execution and return false
.
Attempt to run the above code snippet in any browser that supports JavaScript; it will display the result below.
Output:
false
Access the demo here.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedInRelated Article - JavaScript Array
- How to Check if Array Contains Value in JavaScript
- How to Create Array of Specific Length in JavaScript
- How to Convert Array to String in JavaScript
- How to Remove First Element From an Array in JavaScript
- How to Search Objects From an Array in JavaScript
- How to Convert Arguments to an Array in JavaScript