How to Change Text Colour Using JavaScript
Kushank Singh
Feb 02, 2024
JavaScript
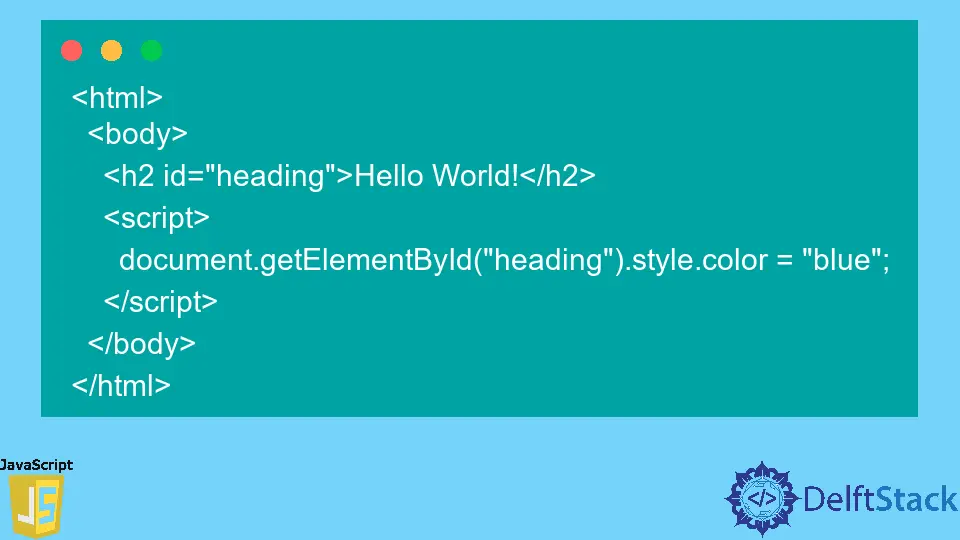
JavaScript and HTML work together to maintain the front-end of many web based applications.
We will learn how to change the text color using JavaScript in this article.
HTML DOM allows JavaScript to change the style of HTML elements. We can use the style.color
property.
This property allows us to set the color of the element or return the current color.
Check the code given below.
<html>
<body>
<h2 id="heading">Hello World!</h2>
<script>
document.getElementById("heading").style.color = "blue";
</script>
</body>
</html>
We altered the color of the <h2>
element in the above example. We accessed the element using its ID heading
in the getElementbyID()
method. Then we used the style.color
property to set the color to blue.
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe