How to Change the Page Title in JavaScript
-
Use the
document.title
Property to Change the Page Title in JavaScript -
Use the
querySelector()
Method to Change the Page Title in JavaScript
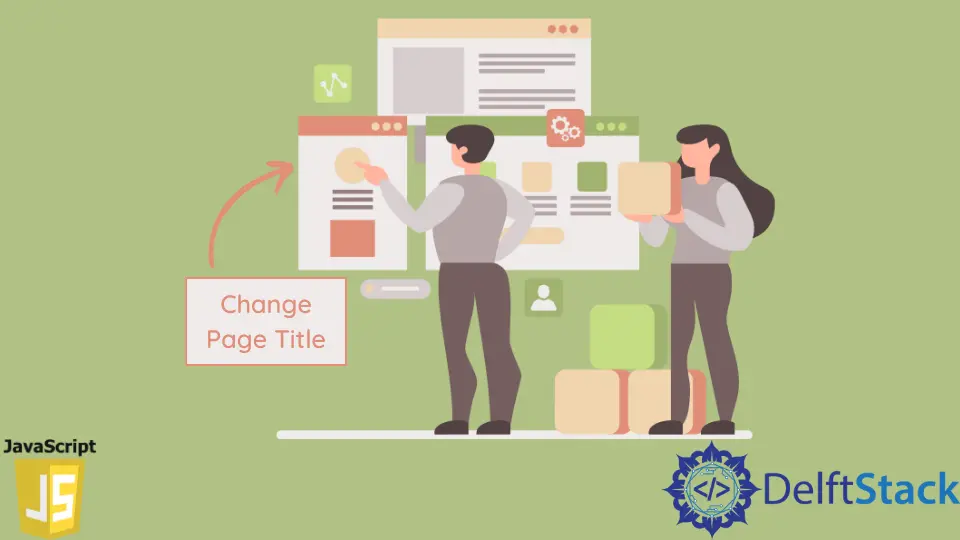
This article will discuss modifying a web page’s title in JavaScript dynamically.
Use the document.title
Property to Change the Page Title in JavaScript
The document.title
attribute is used to set or retrieve the document’s current title. The page’s title can be modified by assigning a new title as a string to this property.
The website’s title will then be changed to the selected title.
Syntax:
newPageTitle = 'Title changed!';
document.title = newPageTitle;
Example:
<!DOCTYPE html>
<html>
<head>
<title>
Dynamically change a web
page's title
</title>
</head>
<body>
<b>
Dynamically change a web
page's title
</b>
<p>
Click on the button to change the page
title to: "title changed!"
</p>
<button onclick="changePageTitle()">
Change Page Title
</button>
<script type="text/javascript">
function changePageTitle() {
newPageTitle = 'title changed!';
document.title = newPageTitle;
}
</script>
</body>
</html>
Output before clicking the button:
Output after clicking the button:
Use the querySelector()
Method to Change the Page Title in JavaScript
We can use the document.querySelector()
method to pick elements in a document.
The title element can be chosen by giving the title element a selector parameter and retrieving the page’s main title element.
An element’s textContent
attribute returns the text content of a particular location. The page’s title can be modified by setting the textContent
property to the required new title as a string.
Syntax:
newPageTitle = 'title changed!';
document.querySelector('title').textContent = newPageTitle;
Example:
<!DOCTYPE html>
<html>
<head>
<title>
Dynamically change a web
page's title
</title>
</head>
<body>
<b>
Dynamically change a web
page's title
</b>
<p>
Click on the button to change the page
title to: "title changed!"
</p>
<button onclick="changePageTitle()">
Change Page Title
</button>
<script type="text/javascript">
function changePageTitle() {
newPageTitle = 'title changed!';
document.querySelector('title').textContent = newPageTitle;
}
</script>
</body>
</html>
Output before clicking the button:
Output after clicking the button:
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedIn