How to Change Label Text Using JavaScript
-
What Is
<label>
in JavaScript -
Use
.innerHTML
to Change Label Text Using JavaScript -
Use jQuery’s
.text()
Method to Change Label Text Using JavaScript
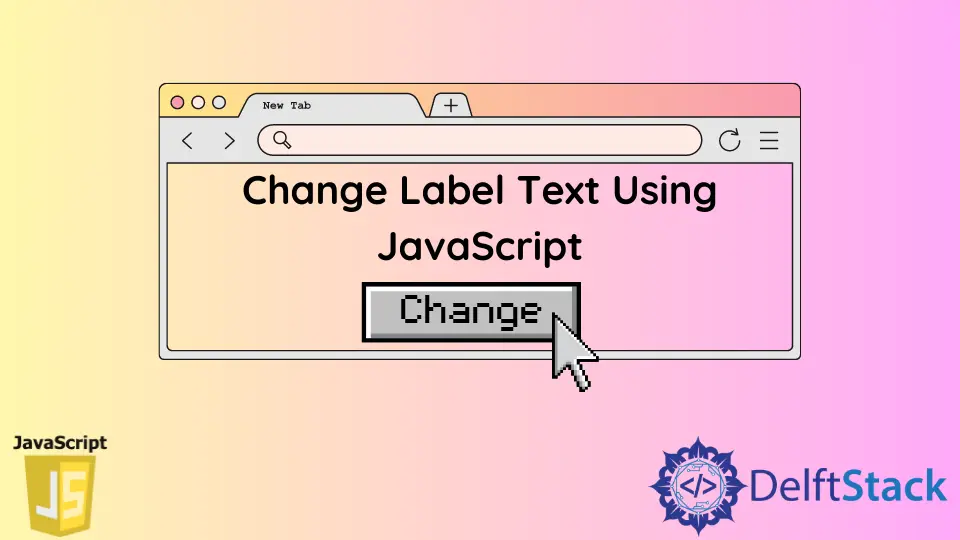
This tutorial educates how to change label text using JavaScript. We’ll be using .innerHTML
and jQuery’s text()
method to meet the goal for this tutorial.
So, let’s start!
What Is <label>
in JavaScript
The label (<label>
) is an HTML tag that we can use to define the caption of an element or text label for an <input>
element. You can find different uses of the label (<label>
) tag here.
Use .innerHTML
to Change Label Text Using JavaScript
HTML Code:
<!DOCTYPE html>
<html>
<head>
<title>
Change the Text of A label in JavaScript
</title>
</head>
<body>
<label id = "label">
Change this text by clicking on the button
</label>
<br>
<button onclick="changeLabelText()">
Click Here
</button>
</body>
</html>
JavaScript Code:
function changeLabelText() {
document.getElementById('label').innerHTML =
'The text of this label has been changed';
}
OUTPUT (before pressing the button Click Here
):
OUTPUT (after pressing the button Click Here
):
Here, we are using the .innerHTML
property and assigning a new text displayed after clicking on the Click Here
button. The .innerHTML
returns the string inside the HTML element and HTML/XML markup that resides within the string, for instance, space and line breaker etc.
We use this property to see what is in the HTML element - including any line break, spacing, formatting irregulars, etc. The .innerHTML
property returns as HTML entities if the text has characters >
, <
, or &
.
We can change the JavaScript code a bit and can switch between the old text and new text after pressing the button Click Here
. The updated JavaScript code will be as follows:
function changeLabelText() {
var element = document.getElementById('label');
if (element.innerHTML == 'Change this text by clicking on the button')
element.innerHTML = 'The text of this label has been changed';
else
element.innerHTML = 'Change this text by clicking on the button';
}
OUTPUT:
Use jQuery’s .text()
Method to Change Label Text Using JavaScript
HTML Code:
<!DOCTYPE html>
<html>
<head>
<title>
Change the Text of A label in JavaScript
</title>
</head>
<body>
<label id = "label">
Change this text by clicking on the button
</label>
<br>
<button onclick="changeLabelText()">
Click Here
</button>
</body>
</html>
JavaScript Code:
function changeLabelText() {
var label_text = $('#label').text();
if (label_text === 'Change this text by clicking on the button')
$('#label').text('The text of this label has been changed');
else
$('#label').text('Change this text by clicking on the button');
}
OUTPUT:
Here, we get the text using jQuery’s text()
method and save it into the label_text
variable. Further, we check the value and type of label_text
and compare it with Change this text by clicking on the button
using ===
operator.
If it is true
, pass the new text to the text()
method; otherwise, it is not.