How to Change Image Source JavaScript
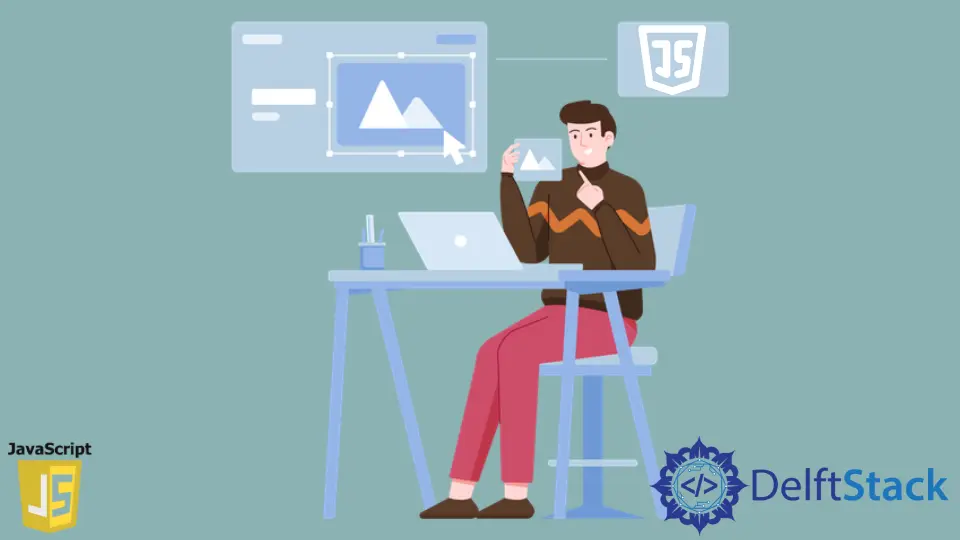
This tutorial will introduce how to change the source or src
of an image using the src
property in JavaScript.
Change the Source of an Image Using the src
Property in JavaScript
To change the source or src
of an image, you need to add an id
or class
to the image tag. You can get the image element using the name of the id
or class
, and you can change the source or src
of the image using the src
property. See the example code below.
document.getElementById('myImageId').src = 'newSource.png';
In the above code, we use the getElementById()
function to get the element using its id
and then changing its source using the src
property. You can also use the name of the class to get the element using the getElementByClassName()
function. You can put the id
of the image tag in the place of the myImageId
and the new source or src
of the image in the place of the newSource.png
in the code.