JavaScript CDATA
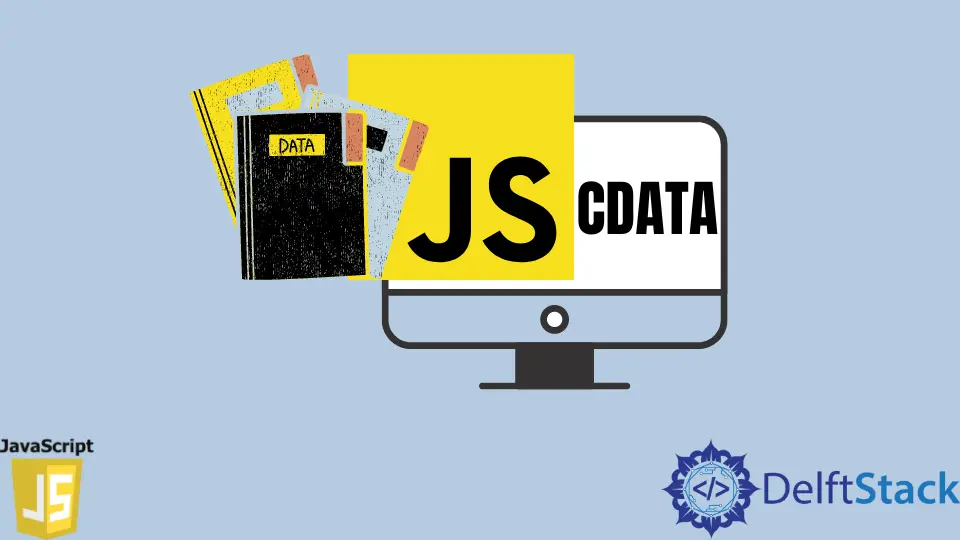
This article will teach about the CDATA
section in JavaScript. First, we will learn what the CDATA
is and how it works.
We will also know where and when to use the CDATA
section in JavaScript.
What Is a CDATA
Section
The CDATA
section object represents a CDATA
in a document. It contains text that a parser will not parse.
The tags inside a CDATA
will not be treated as markup and entities. We use the CDATA
to mark a section of an XML document so that the XML parser interprets it only as character data and not markup data.
As we learned what the CDATA
section is, now we will discuss when it is necessary to use the CDATA
section in JavaScript.
When Is Using a CDATA
Section Necessary in JavaScript
Sometimes JavaScript code contains a lot of "<
or >"
or "&"
characters. When we use symbols like <
and >
or &
to compare two integers etc., within JavaScript.
To avoid parsing these symbols <
or >
and &
we use the CDATA
section tag (<script>....</script>
) in JavaScript. The parser ignores everything inside a CDATA
section.
To ensure that the XHTML
validation works correctly on JavaScript that we use in the HTML page, we use the CDATA
section tag. We use the section tag like this.
Example code:
<script>
//<![CDATA[
document.write("> or <");
//]]>
</script>
Using the CDATA
section tag within a script is necessary to avoid XML errors in XHTML validation. The CDATA
tells the browser to display the text used in JavaScript.
When the browsers treat the markup as XML, we use the following CDATA
tag code in JavaScript.
Example code:
<script>
<![CDATA[
...code...
]]>
</script>
When the browsers treat the markup as HTML, we use the following CDATA
tag code in JavaScript.
Example code:
<script>
...code...
</script>
So this is how we use the CDATA
section tag in JavaScript.
We hope you find this article helpful in understanding the use of the CDATA
in JavaScript.
My name is Abid Ullah, and I am a software engineer. I love writing articles on programming, and my favorite topics are Python, PHP, JavaScript, and Linux. I tend to provide solutions to people in programming problems through my articles. I believe that I can bring a lot to you with my skills, experience, and qualification in technical writing.
LinkedIn