How to Call Python From JavaScript
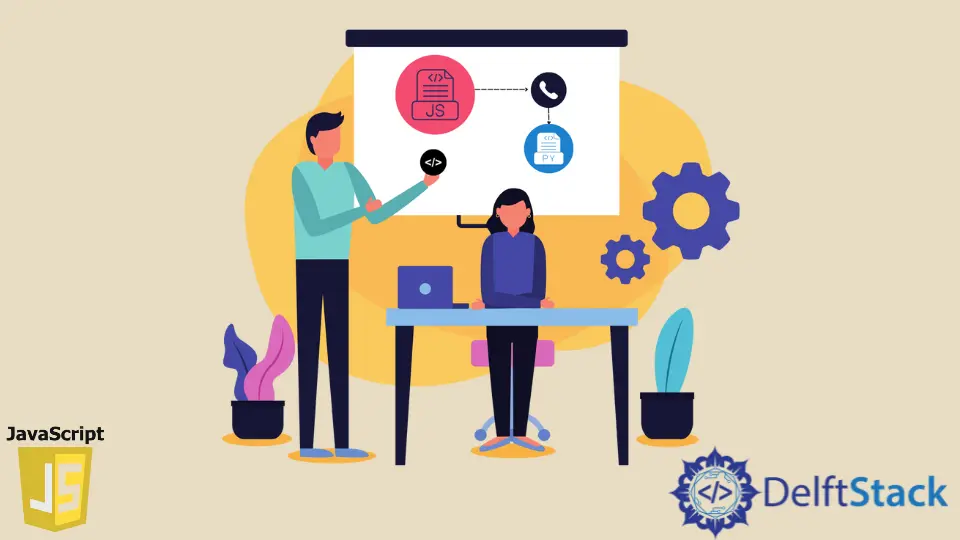
Calling server file from the frontend (HTML) is a common use case for every dynamic website. All the dynamic website connects with the server via various methods.
This article teaches how to call Python from JavaScript.
Use ajax
to Call Python From JavaScript
AJAX stands for Asynchronous JavaScript and XML. It utilizes the XMLHttpRequest
object to communicate with servers.
It can send and receive information in numerous formats, including HTML, XML, JSON, and text files. The most interesting feature of AJAX is its “asynchronous” nature, which means that it can communicate with the server, exchange data, and refresh the page while not having to refresh the page.
The two main features of AJAX that allow you to perform are below.
- Make asynchronous requests to the server without reloading the HTML/JavaScript page.
- Receive data from the server and process it per the client’s requirement.
Syntax:
$.ajax({
url: 'SERVER_URL',
data: {param: text},
type: 'HTTP_METHOD',
}).done(function() {
/* Process the data */
});
- The
ajax
method accepts the URL as an input parameter, a string containing the URL to which the request is sent. - Data is a
JSON
object which contains the parameters to send to the server. If theHTTP
method is a method that cannot have an entity-body, such asGET
, the data is appended to the URL. type
is theHTTP
method used to request the server..done
is an alternative construct to the successful callback option, and please refer todeferred.done()
for implementation details.
Example:
$.ajax({
type: 'POST',
url: '~/helloWorld.py',
data: {
param: 'hello world',
}
}).done((o) => {console.log(o)});
In the above code, we make POST
requests to the server with the parameter as hello world
. It will call the helloWorld.py
Python file, which will accept the input parameters and process the data.
It will then return the response to the client.
Output:
Thank you.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn