How to Call a JavaScript Function Into an HTML Body
Valentine Kagwiria
Feb 02, 2024
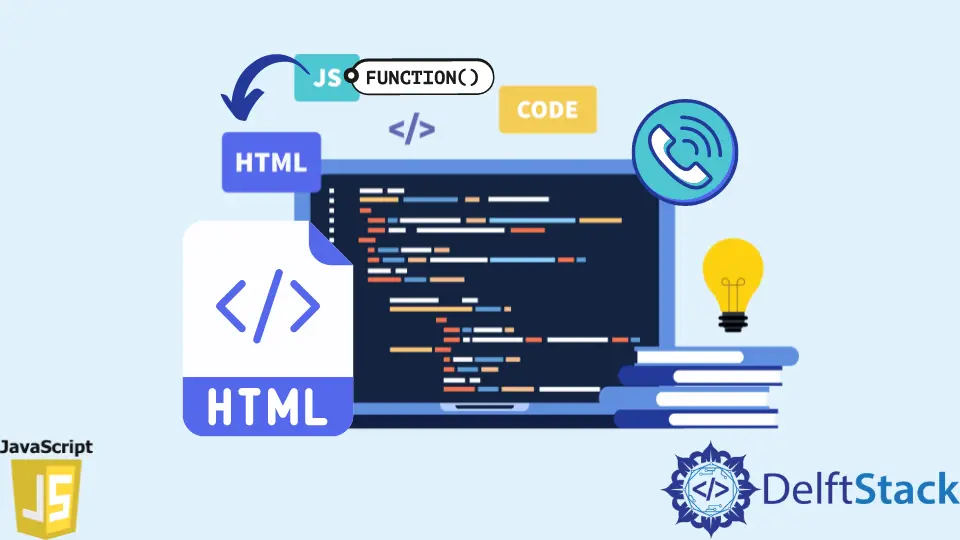
This article explains how to call a JavaScript function to execute in an HTML body by using the <script>
tag. It also shows how to create the function in a JavaScript file and link it to the HTML file.
Call a JavaScript Function in a HTML <script>
Tag
If you are using a JavaScript function, one of the best places to call it in an HTML body is in a