How to Calculate the Percentage in JavaScript
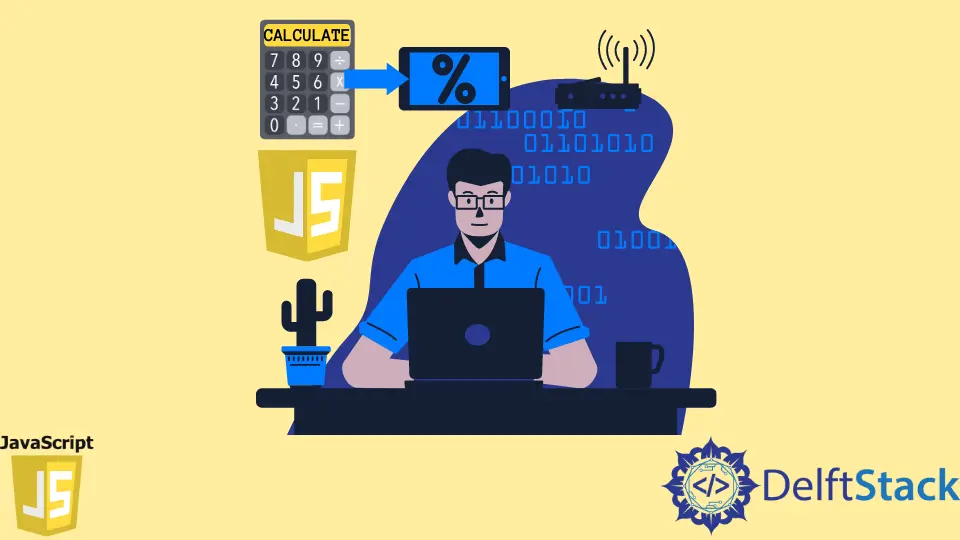
This article will tackle how to calculate the percentage of any number using JavaScript.
Calculate the Percentage in JavaScript
It is very easy to get the percentage of any number in JavaScript. To get the percentage of any number in basic math, we must multiply the desired percentage percent by that number.
In the below JavaScript code example, we create a function called calPercnt
. In that function, we used a simple formula to get the 20% of 500.
In the formula, we divided 20 by 100, equal to 0.2. We then multiplied 0.2 by 500, which gives us 100, which is 20% of 500.
Example Code:
function calPercnt(num, percentage) {
const result = num * (percentage / 100);
return parseFloat(result.toFixed(2));
}
const percntVal = calPercnt(500, 20);
console.log(percntVal);
Output:
100
This is one way to calculate the percentage of a number in JavaScript. We can also use a custom JavaScript function to calculate the percentage of a number.
Use Custom JavaScript Function to Calculate the Percentage
It is one of the easiest and short methods used to calculate the percentage of any number in JavaScript. This custom function takes two parameters: the percentage
that we want to calculate and the number
of which we want to get the percentage.
We call the function calcPercnt
in the console.log
, and at runtime, we give two parameters that we want to calculate the percentage of.
In the code example, we gave the console.log
(1000, 20) that will calculate the 20% of 1000.
These are the few examples that the function being used to calculate the percentages console.log(calcPercnt(1000, 30));
, console.log(calcPercnt(1000, 40));
, console.log(calcPercnt(1000, 45));
, console.log(calcPercnt(1000, 50));
, console.log(calcPercnt(100, 10));
.
We give parameters in the console.log
section in the custom function. We get the percentage of a number we want to calculate in the output.
Example Code:
function calcPercnt(percent, num) {
return (percent / 100) * num;
}
console.log(calcPercnt(1000, 20));
console.log(calcPercnt(1000, 30));
console.log(calcPercnt(1000, 40));
console.log(calcPercnt(1000, 45));
console.log(calcPercnt(1000, 50));
console.log(calcPercnt(100, 10));
Output:
200
300
400
450
500
10
In the output above, we get 200, 20% of 1000. Then we get 300, which is 30% of 1000, and then we get 400.
This custom function helps us find multiple percentages of numbers at once by giving the values into parameters.
My name is Abid Ullah, and I am a software engineer. I love writing articles on programming, and my favorite topics are Python, PHP, JavaScript, and Linux. I tend to provide solutions to people in programming problems through my articles. I believe that I can bring a lot to you with my skills, experience, and qualification in technical writing.
LinkedIn