Bitwise XOR Operator in JavaScript
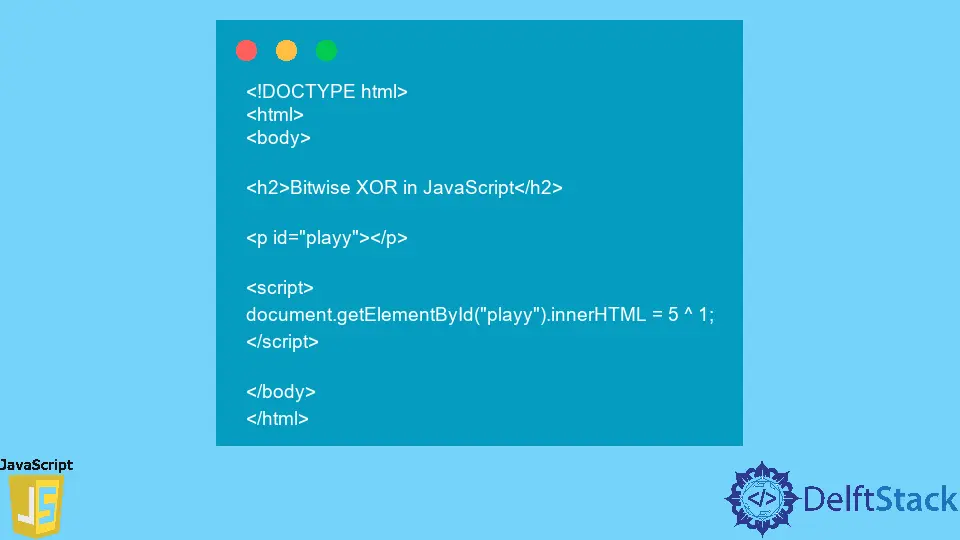
XOR
is a bitwise Boolean operator which only takes true or false operands and returns only true or false. Consequently, JavaScript does not have a logical XOR
operator since JavaScript traces its lineage back to the C language, and C does not have a logical XOR
operator.
Use the XOR (^)
Operator in JavaScript
The XOR (^)
operator can make a bitwise comparison of two numbers, but this does not assist when you wish to acquire the result of an XOR
of two expressions that do not yield a number. Considering JavaScript has an XOR
operator existing, it would indeed be excellent to utilize it.
It is feasible, but the operator anticipates to be provided numbers. So all you have to do is offer it different integers depending on the phrases that need to be evaluated.
Make sure that numbers are the same as each other when they assess to true
and 0
when considered false
. In that manner, when the variables are evaluated, the resulting value will be that number.
The table above shows that it returned a value of 1
if two operands are different and 0
for the same operand.
Syntax:
a ^ b
Example:
<!DOCTYPE html>
<html>
<body>
<h2>Bitwise XOR in JavaScript</h2>
<p id="playy"></p>
<script>
document.getElementById("playy").innerHTML = 5 ^ 1;
</script>
</body>
</html>
When XOR
is performed, the binary result is 00000400
, equal to the decimal number 4
.
Output:
4
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedIn