Array vs Object Declaration in JavaScript
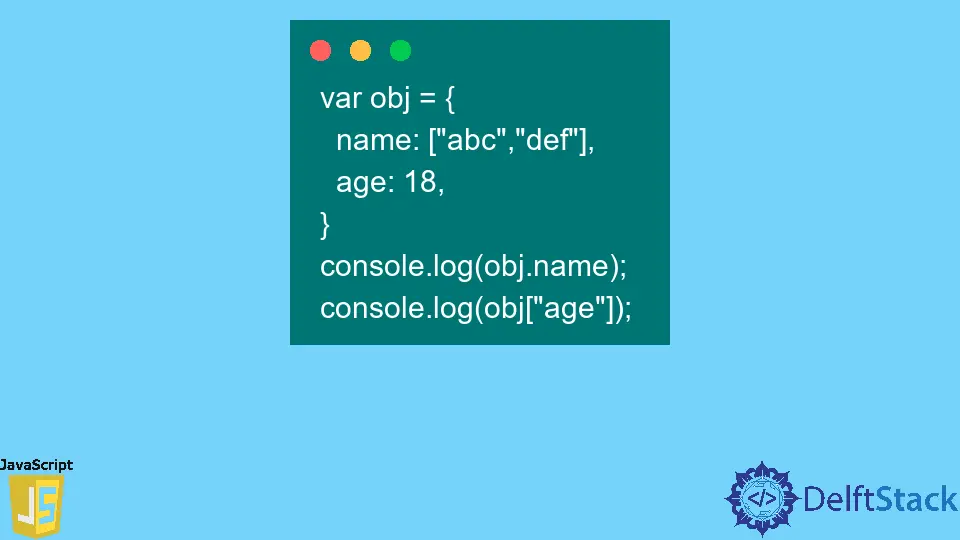
Arrays and objects are both mutable and can store multiple values. They both are considered a vital part of JavaScript.
We will learn about the difference between array and object declaration in JavaScript in this article.
Arrays are used when we store multiple values of a single variable, while an object can hold multiple variables with their values.
An array can also be considered as an object and has most of the object functionalities. It has some additional features like length
, pop()
, slice()
, etc.
To declare arrays, we will use the square brackets []
.
See the following code.
var name = ['abc', 'def']
console.log(name)
Output:
["abc","def"]
In the above example, we declared an array called name
and printed its contents. Note that elements in an array are stored at specific indexes, which can be used to access them.
On the other hand, an object lets us associate name
with a value as a pair. We can use the keys to access values from an object.
To declare an object, we will use the curly braces {}
.
For example,
var obj = {
name: ['abc', 'def'],
age: 18,
} console.log(obj.name);
console.log(obj['age']);
Output:
["abc","def"]
18
The above example should clear things up. We created an object called obj
. One of the pairs contains an array. We were able to access the elements using their keys.
Related Article - JavaScript Object
- How to Search Objects From an Array in JavaScript
- How to Get the Object's Value by a Reference to the Key
- How to Find Object in Array by Property Value in JavaScript
- How to Print Objects in JavaScript
- How to Destroy Object in JavaScript
- Nested Objects in JavaScriptn
Related Article - JavaScript Array
- How to Check if Array Contains Value in JavaScript
- How to Create Array of Specific Length in JavaScript
- How to Convert Array to String in JavaScript
- How to Remove First Element From an Array in JavaScript
- How to Search Objects From an Array in JavaScript
- How to Convert Arguments to an Array in JavaScript