Array Filter in JavaScript
Jagathish
Oct 12, 2023
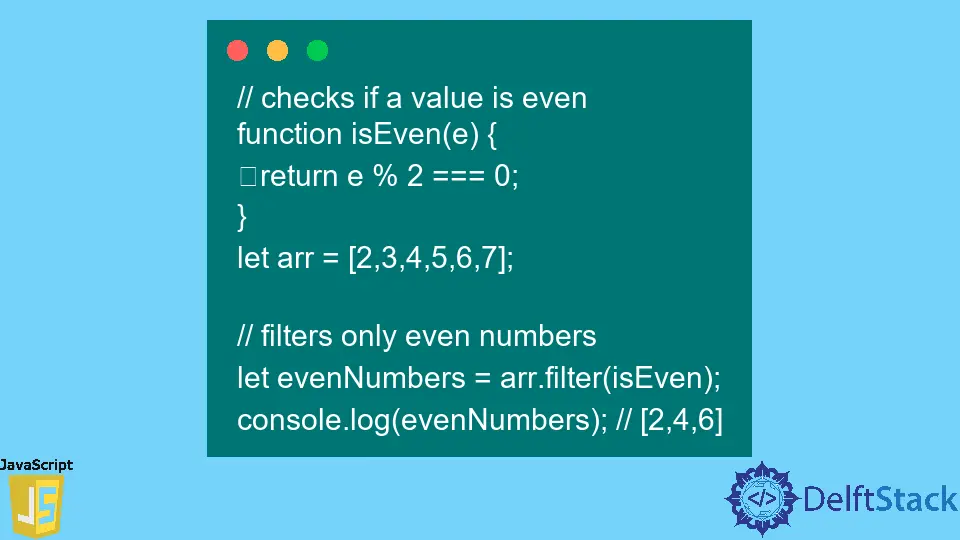
The filter()
method in JavaScript returns a new array containing all elements passed the specified function’s test. This post will discuss how to get array elements that match a certain condition.
Using the isEven()
Function in JavaScript
We have numbers in an array, and we need to select all the even
elements.
Example:
// checks if a value is even
function isEven(e) {
return e % 2 === 0;
}
let arr = [2, 3, 4, 5, 6, 7];
// filters only even numbers
let evenNumbers = arr.filter(isEven);
console.log(evenNumbers); // [2,4,6]
Output:
input : [2,3,4,5,6,7]
output : [2,4,6]
In the above code:
- Created a function
isEven
, which will take one argument. This function returnstrue
if an argument is aneven
number. Otherwise,false
will be returned. - Created an array
arr
and called thefilter
method of thearr
withisEven
function as an argument. - The
filter
method will loop through all the array elements and execute theisEven
method for each array element. Thefilter
method will select all the elements for which theisEven
method returns the value of2,4,6
.
Using the isPalindrome()
Function in JavaScript
We have an array of strings, and we need to select all the strings which are palindrome
(a string of characters that reads the same backward as forward, e.g., mom
, dad
, madam
).
Example:
// checks if a string is palindrome
function isPalindrome(str) {
// split each character of the string to an array and reverse the array and
// join all the characters in the array.
let reversedStr = str.split('').reverse().join('');
return str === reversedStr;
}
let arr = ['dad', 'god', 'love', 'mom']
// filters only palindrome string
let palindromeArr = arr.filter(isPalindrome);
console.log(palindromeArr); // ['dad', 'mom']
Output:
input : ['dad', 'god', 'love', 'mom']
output : ['dad', 'mom']
In the above code:
- Created a function
isPalindrome
, which will take one string argument. This function returnstrue
if the argument is apalindrome
. Otherwise,false
will be returned. - Created an array
arr
and called thefilter
method of thearr
withisPalindrome
function as an argument. - The
filter
method will loop through all the array elements and execute theisPalindrome
method for each array element. Thefilter
method will select all the elements for which theisPalindrome
method returns the value'dad'
,'mom'
.
Related Article - JavaScript Array
- How to Check if Array Contains Value in JavaScript
- How to Create Array of Specific Length in JavaScript
- How to Convert Array to String in JavaScript
- How to Remove First Element From an Array in JavaScript
- How to Search Objects From an Array in JavaScript
- How to Convert Arguments to an Array in JavaScript