How to Add Properties to JavaScript Object
- Use Square Bracket Notation to Add Properties to JavaScript Object
- Use Dot Notation to Add Properties to JavaScript Objects
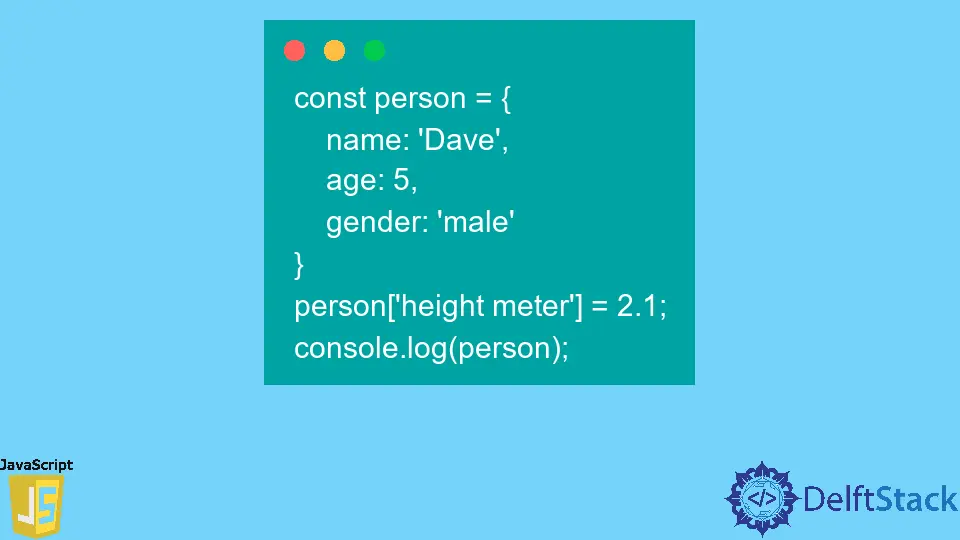
This tutorial introduces how to add properties to JavaScript objects.
Use Square Bracket Notation to Add Properties to JavaScript Object
Square bracket notation is equivalent to Dot Notation with one major difference. We can use it to add properties with more than one word in their names. For example, we can use square bracket notation like person["one more"]
but can not do it using the dot notation.
const person = {
name: 'Dave',
age: 5,
gender: 'male'
} person['height meter'] = 2.1;
console.log(person);
Output:
{name: "Dave", age: 5, gender: "male", height: 2.1}
In the above code, we have used the square bracket notation person["height meter"]
to add height to the Object person.
Use Dot Notation to Add Properties to JavaScript Objects
const person = {
name: 'Dave',
age: 5,
gender: 'male'
} person.height = 2.1;
console.log(person);
Output:
{name: "Dave", age: 5, gender: "male", height: 2.1}
In the above code, we have used the Dot Notation person.height
to add height to the Object person.
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn