How to Add Elements at the Beginning of an Array in JavaScript
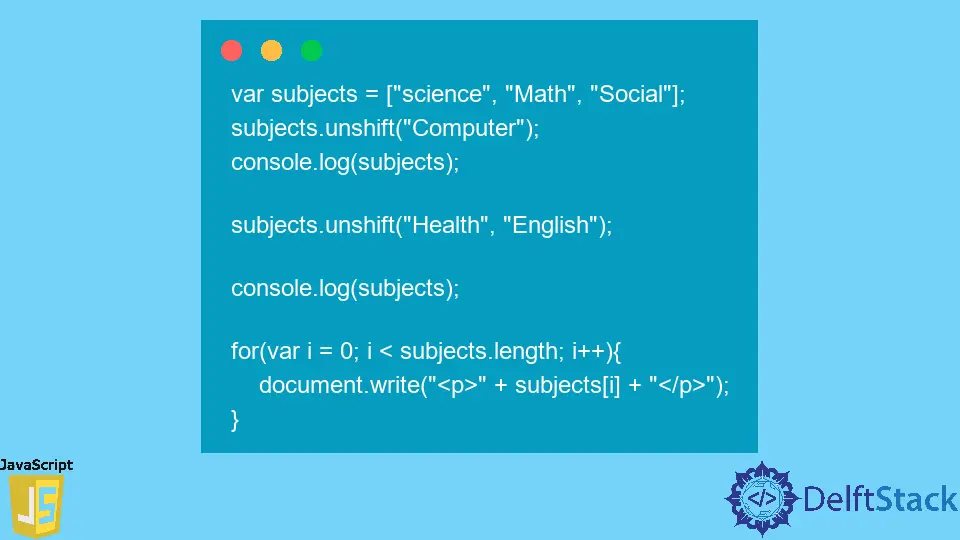
In JavaScript, you may use the unshift()
method to quickly add elements or values at the beginning of an array.
Use the unshift()
Method to Add Elements at the Beginning of an Array in JavaScript
The unshift()
method adds the specified values to the start of an array-like object. This method is the inverse of the push()
method, which adds elements to an array’s end.
Both approaches, however, return the array’s updated length.
Syntax:
unshift(object0)
unshift(object0, object1)
unshift(object0, object1, /* ... ,*/ objectN)
unshift()
is designed to be generic. Array-like objects can be called or applied to this method.
Things without a length property - which represents the latest series of zero-based numerical properties - might not behave in any meaningful way.
If multiple elements are supplied as arguments, they will be added in chunks at the beginning of the object, in the same order as they were passed as parameters.
As a result, calling unshift()
once with n parameters or calling it n times with one argument (for example, in a loop) do not produce the same results.
The following example demonstrates how to start an array with a single or more entry.
Example:
var subjects = ['science', 'Math', 'Social'];
subjects.unshift('Computer');
console.log(subjects);
subjects.unshift('Health', 'English');
console.log(subjects);
for (var i = 0; i < subjects.length; i++) {
document.write('<p>' + subjects[i] + '</p>');
}
Output:
["Computer", "science", "Math", "Social"]
You can also run the code.
In the above example, variables named subjects
are created, and then a new element is added to the subject at the front using the unshift()
method.
Again, if two subjects are added to the array at the front with the help of the unshift()
method.
Output:
["Health", "English", "Computer", "science", "Math", "Social"]
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedInRelated Article - JavaScript Array
- How to Check if Array Contains Value in JavaScript
- How to Create Array of Specific Length in JavaScript
- How to Convert Array to String in JavaScript
- How to Remove First Element From an Array in JavaScript
- How to Search Objects From an Array in JavaScript
- How to Convert Arguments to an Array in JavaScript