How to Add Attribute to DOM Element in JavaScript
-
Set Attribute to a DOM Element Using the
setAttribute
Method -
Set Attribute to a DOM Element Using the
setAttributeNode
Method
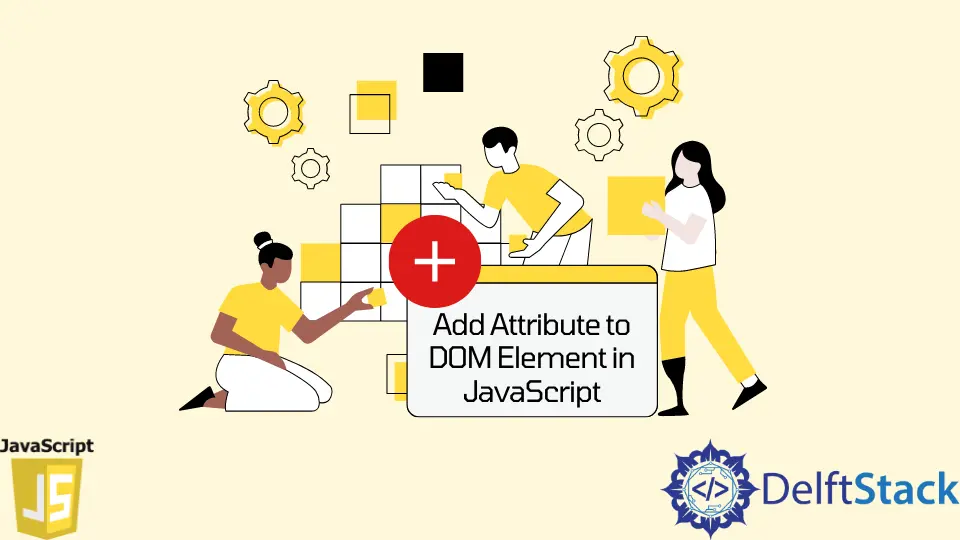
This tutorial teaches how to add an attribute to a DOM element. We can add an attribute to a DOM element using two methods.
First, is the setAttribute(name, value)
and second is the setAttributeNode(attribute_node)
.
Set Attribute to a DOM Element Using the setAttribute
Method
The setAttribute
method will:
- Add a new attribute to the DOM element if the specified attribute is not present.
- Update the value of the attribute of the DOM element if the specified attribute is already present.
This method takes the attribute name and value as an argument.
<div id="div" name="div_ele" > Div </div>
JavaScript code to set an attribute using the setAttribute
method.
let divElement = document.getElementById('div');
divElement.setAttribute('order', 1);
divElement.setAttribute('name', 'DIV');
After executing the above code, the div element will have a new attribute order
with value 1
, and the value of the attribute name
will be updated from div_ele
to DIV
.
<div id="div" name="DIV" order="1"> Div </div>
Set Attribute to a DOM Element Using the setAttributeNode
Method
The functionality of setAttributeNode
method is smiliar to setAttribute
method. The only difference is setAttributeNode
method takes the Attr
node as an argument.
<div id="div" name="DIV" order="1"> Div </div>
JavaScript code to set attribute using setAttributeNode
method
let divElement = document.getElementById('div');
let orderAttrNode = document.createAttribute('order');
orderAttrNode.value = '2';
divElement.setAttributeNode(orderAttrNode);
let testAttrNode = document.createAttribute('test');
testAttrNode.value = 'test_div';
divElement.setAttributeNode(testAttrNode);
After executing the above code, the div element will have a new attribute test
with value test_div
, and the value of the attribute order
will be updated from 1
to 2
.
<div id="div" name="DIV" order="2" test="test_div"> Div </div>
To test the validity of the codes above, you may visit this link.