How to Convert XML to JSON in Java
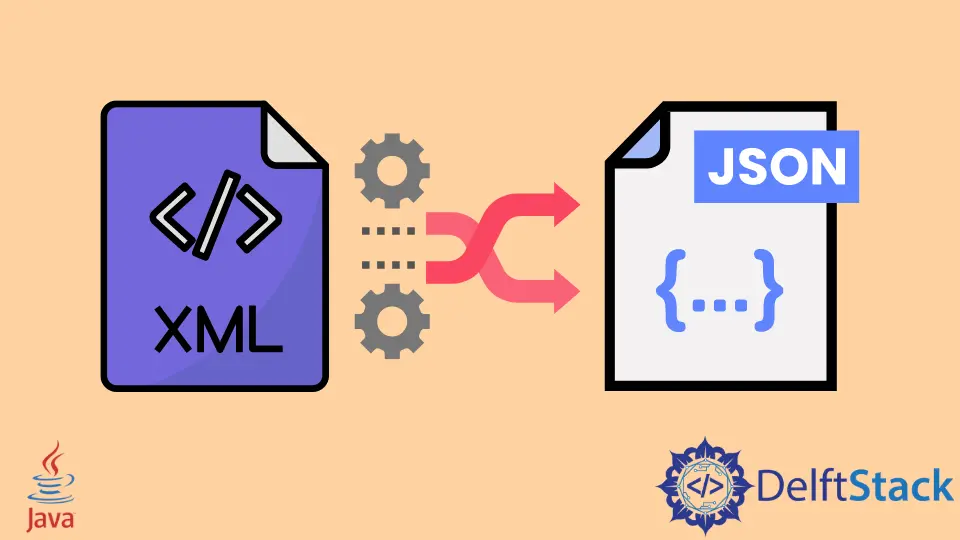
This article will introduce how to convert an XML file into JSON in Java. XML is Extensible Markup Language
, and any text that you send through email is received as an XML and later converted into JSON to store in a database. Take a look at the following example.
XML Representation
The following code is an example of an XML string. Let’s say, somebody sent you any particular data by email. You will receive it as an XML. It will be converted into readable text for you later on. In order to do that in Java, you need to import org.json
. Take a look at the following example.
import java.util.*;
import org.json.*;
public class Main {
public static void main(String args[]) {
String examplexml = // example xml
"<?xml version=\"1.0\" ?><root><test attribute=\"Name\">Bill Gates</test><test attribute=\"RollNumber\">01</test></root>";
System.out.println(examplexml); // printing Example of XML
}
}
Convert XML to JSON in Java
The above XML string is converted to Json in Java using the try...catch
method. In the JSON library, there’s a method known as toJSONObject()
. In the parenthesis ()
you will pass the object of XML string, in this case, examplexml
. Learn more about JSON in Java here. In order to read the xml string, we’ll be converting JSON into string. Take a look at the following code.
System.out.println("JSON IS");
try {
JSONObject json = XML.toJSONObject(examplexml);
String jsonString = json.toString(4);
System.out.println(jsonString);
} catch (Exception e) {
}
}
The code will give the following output. Use the following command to run this type of program.
javac -cp "org.json.jar" Main.java
java -cp "org.json.jar" Main.java
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedInRelated Article - Java JSON
- How to Pretty-Print JSON Data in Java
- How to Serialize Object to JSON in Java
- How to Convert JSON Data to String in Java
- How to Deserialize JSON in Java
- How to Handle JSON Arrays in Java
- How to Convert JSON to Java object