What Does Instantiate Mean in Java
-
Use the
new
Keyword to Instantiate a Class in Java - Difference Between Initialization and Instantiation in Java
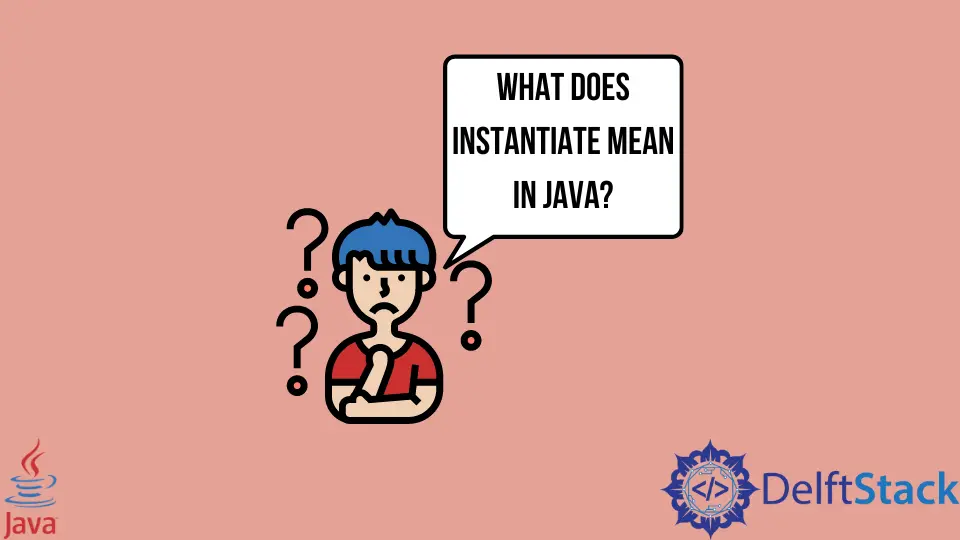
This tutorial goes through the concept of instantiation in Java. We use objects in Java because it is an object-oriented programming language.
The points below will show what instantiate means in Java and how it differs from initialization.
Use the new
Keyword to Instantiate a Class in Java
We use the concept of instantiation in Java when we create a new object. To create a new object, we use the keyword new
that instantiates an object and returns a reference to the object.
An object is a class blueprint, and when we want to access that class and its properties, we need to instantiate the class’s object.
In the following program, we have two classes: the JavaExample
with the main()
method and another class called ExampleClass
without anything inside.
To instantiate the ExampleClass
class, we use the new
keyword and its constructor ExampleClass()
to initialize it.
The memory is allocated using the new
keyword to instantiate a class to create an object. The reference is returned that we store in a variable exampleClass
, also called an instance of that class.
public class JavaExample {
public static void main(String[] args) {
ExampleClass exampleClass = new ExampleClass();
}
}
class ExampleClass {}
Difference Between Initialization and Instantiation in Java
Instantiation is sometimes confused by another term in Java that is initialization.
- Instantiation - We create an object using the
new
keyword that returns an instance of a class, and the memory is allocated to the object. - Initialization - We put value in the memory allocated during instantiation.
To understand the topic better, let’s see an example.
In the code below, we use the new
keyword to instantiate a class and create its object; instantiation happens.
When the new
keyword is used with the class’s constructor like below, we use the new ExampleClass()
called initialization. We put the reference in the instance of ExampleClass
.
ExampleClass exampleClass = new ExampleClass(10, "Ten");
Another basic example is shown below.
Here we declare two variables, and in the class’s constructor, we insert the values passed to the constructor. It is called initialization.
class ExampleClass {
int value1;
String value2;
ExampleClass(int value1, String value2) {
this.value1 = value1;
this.value2 = value2;
}
}
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn