How to Fix The Superclass Javax.Servlet.Http.HttpServlet Was Not Found on the Java Build Path
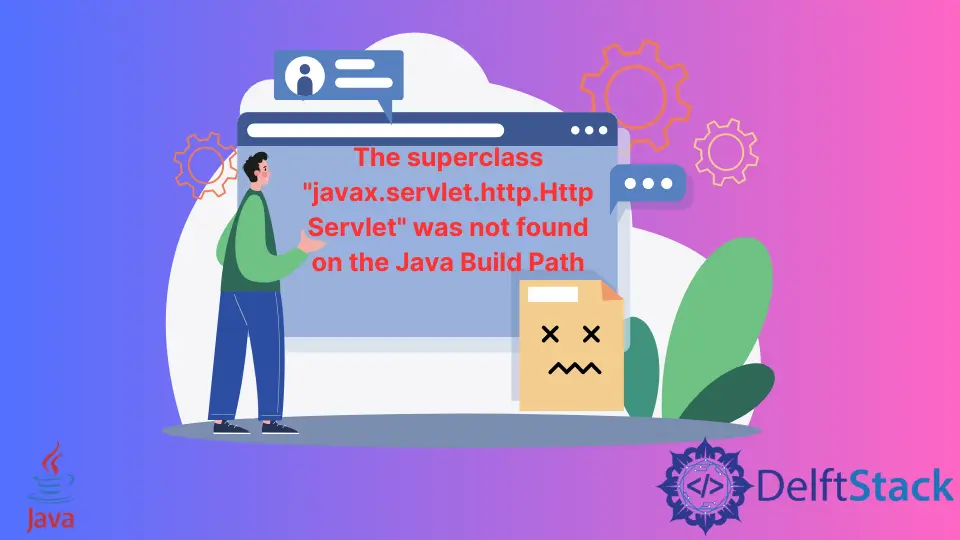
This tutorial demonstrates how to solve the The superclass "javax.servlet.http.HttpServlet" was not found on the Java Build Path
in Java.
The superclass "javax.servlet.http.HttpServlet" was not found on the Java Build Path
in Java
The error The superclass "javax.servlet.http.HttpServlet" was not found on the Java Build Path
occurs when we write our first JSP code in Eclipse, or we are importing an existing Maven project into Eclipse.
This error is because HttpServlet
is not available in the classpath
of the project.
Solution for Eclipse Class Path
We need to add the Apache Tomcat server to the Eclipse IDE to solve this issue. Follow these steps below to solve this issue.
-
Right-click on the project name and open
Properties
. -
Go to
Project Facets
. -
After opening the
Project Facets
, on the right side of the tab, you will seeDetails
andRuntimes
options. ClickRuntimes
. -
Select or check the
Apache Tomcat Server
. -
Click
Apply
, thenOk
.
Following the process above will solve the error The superclass "javax.servlet.http.HttpServlet" was not found on the Java Build Path
.
Solution for Maven/Gradle
Another reason for this error is that when working with Maven or Gradle, we must add the servlet-api
into the Maven or Gradle dependencies. Follow the process below.
-
Download the Java Servlet API.
-
Add the
javax.servlet-api-4.0.1.jar
to your build path. -
Now add the
javax.servlet-api-4.0.1.jar
as a dependency.
For Maven:
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<version>4.0.1</version>
<scope>provided</scope>
</dependency>
For Gradle:
configurations{provided} sourceSets {
main {
compileClasspath += configurations.provided
}
}
dependencies {
provided 'javax.servlet:javax.servlet-api:4.0.1'
}
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn FacebookRelated Article - Java Servlet
Related Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack